There is a lot more involved to what you eventually want to do with the player and the camera, however its probably best to learn some parts first before trying to move on.
I rewrote your code as an example to show you, the clearer its laid out, the easier it will be to see the problems at hand, use the arrow keys to move your players ball around, as you can see the camera does not turn with the player etc.
I would do TDKS tutorials before trying to do anymore with this project, get to know the language first, the more commands you understand the better it will be to solve problems int he future.
Anyway I have tried to structure your code so its easier to manage.
Practice using arrays and explore types, they will allow you to manage a lot of data much easier.
Notice also in this example Im using integer based variables to control your 3d media numbers, this will follow on in practice when you get to types etc..
REM ------------------------------------------------------------------------------------------------------------
REM GAME NAME -
REM DBP VERSION -
REM DATE -
REM GAME VERSION -
REM NOTES -
REM ------------------------------------------------------------------------------------------------------------
REM ------------------------------------------------------------------------------------------------------------
REM SETUP ENVIRONMENT
REM ------------------------------------------------------------------------------------------------------------
`Trying to get the basics down!
Set Window on
Set display mode 1024,768,32
Set window size 1024,768
Set window Title "Under Construction"
hide mouse
sync on : sync rate 60
REM ------------------------------------------------------------------------------------------------------------
REM DECLARE VARIABLES
REM ------------------------------------------------------------------------------------------------------------
REM GND = Ground Object
GND = 1
REM PLROBJ = Player Object
PLROBJ = 2
REM ------------------------------------------------------------------------------------------------------------
REM LOAD 2D MEDIA
REM ------------------------------------------------------------------------------------------------------------
LoadGroundTexture()
REM ------------------------------------------------------------------------------------------------------------
REM LOAD 3D MEDIA
REM ------------------------------------------------------------------------------------------------------------
`Attempting to make the ground and texture it
Make Object Box GND,100,5,100
Position Object GND,0,-5,0
`Texture Object GND,GND
color object GND,RGB(0,155,0)
`Attempting to make player manually
Make Object Sphere PLROBJ,10
Position Object PLROBJ,0,5,0
REM ------------------------------------------------------------------------------------------------------------
REM PRE MAIN LOOP
REM ------------------------------------------------------------------------------------------------------------
REM ------------------------------------------------------------------------------------------------------------
REM START MAIN LOOP
REM ------------------------------------------------------------------------------------------------------------
disable escapekey : while escapekey()=0
REM ------------------------------------------------------------------
REM PLAYER CONTROLS
REM ------------------------------------------------------------------
if leftkey() = 1
turn object left PLROBJ,1
endif
if rightkey() = 1
turn object right PLROBJ,1
endif
if upkey() = 1
move object PLROBJ,1
endif
REM ------------------------------------------------------------------
REM UPDATE CAMERA TO THE PLAYERS LOCATION
REM ------------------------------------------------------------------
position camera object position x(PLROBJ),object position y(PLROBJ)+20,object position z(PLROBJ)-50
point camera object position x(PLROBJ),object position y(PLROBJ),object position z(PLROBJ)
REM ------------------------------------------------------------------
REM UPDATE TEXT LAST IN MAIN LOOP AFTER EVERYTHING ELSE
REM ------------------------------------------------------------------
`Putting the Scancode on the screen somewhere
Text 1,1,"scancode : "+STR$(ScanCode())
Text 1,16,"fps : "+STR$(screen fps())
REM ------------------------------------------------------------------------------------------------------------
REM END MAIN LOOP - UPDATE SYNC REFRESH
REM ------------------------------------------------------------------------------------------------------------
fastsync : endwhile
REM ------------------------------------------------------------------------------------------------------------
REM CLEANUP MEDIA
REM ------------------------------------------------------------------------------------------------------------
for i = 1 to 100
if object exist(i)=1
delete object i
endif
next i
for i = 1 to 100
if image exist(i)=1
delete image i
endif
next i
REM ------------------------------------------------------------------------------------------------------------
REM TERMINATE PROGRAM
REM ------------------------------------------------------------------------------------------------------------
END
REM ------------------------------------------------------------------------------------------------------------
REM FUNCTIONS AREA
REM ------------------------------------------------------------------------------------------------------------
Function LoadGroundTexture()
`Load bitmap "ground.bmp",1
endfunction
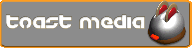