Since you have not supplied enough information if the game is in 2d or 3d i will assume you want it in 3d.
If you have not explored gosubs and functions with TDK's tutorials this will make no sense whatsoever.
I would suggest you do all of TDK's tutorials before getting too deep. You need to learn the concepts of functions / arrays etc, or your going to only hurt your programming ability.
anyway here we go.
rem ------------------------------------------------------
rem Buttons made with 3D Panels
rem indi June 21 2007
rem DBP 6.3 but should work with classic as well
rem ------------------------------------------------------
REM ---------------------------------------------------------------------------------
REM SETUP TEMP ENVIRONMENT
REM ---------------------------------------------------------------------------------
set display mode 1024,768,32
sync rate 60 : sync on : autocam off : randomize timer() : color backdrop rgb(0,0,0)
fontsize = 24 : set text font "Verdana" : set text to bold : set text size fontsize
REM ---------------------------------------------------------------------------------
REM VARIABLES - ARRAYS TO HOLD POSITIONS AND SIZE
REM ---------------------------------------------------------------------------------
dim btn_x(5) : dim btn_y(5)
btn_x(1) = 512 : btn_y(1) = 300
btn_x(2) = 512 : btn_y(2) = 350
btn_x(3) = 512 : btn_y(3) = 400
btn_x(4) = 512 : btn_y(4) = 450
btn_x(5) = 512 : btn_y(5) = 500
dim btn_siz_x(5) : dim btn_siz_y(5)
for i = 1 to 5 : btn_siz_x(i) = 256 : btn_siz_y(i) = 32 : next i
REM ---------------------------------------------------------------------------------
REM MAKE THE 3d MENU BUTTONS
REM ---------------------------------------------------------------------------------
for i = 1 to 5 : Makebtn3D(i) : next i
REM ---------------------------------------------------------------------------------
REM FILL THE ARRAYS
REM ---------------------------------------------------------------------------------
Texture_3D_btn(1,"New Character",1)
Texture_3D_btn(2,"Load Game",2)
Texture_3D_btn(3,"Online Realm",3)
Texture_3D_btn(4,"Options",4)
Texture_3D_btn(5,"Exit Game",5)
REM ---------------------------------------------------------------------------------
REM TEMP VISUAL PARTICLES
REM ---------------------------------------------------------------------------------
make fire particles 1,100,20,0.0,0.0,1.0,1,3,1
` Particle Number, Image Number, Frequency, X, Y, Z, Width, Height, Depth
REM ---------------------------------------------------------------------------------
REM PRE MAIN
REM ---------------------------------------------------------------------------------
position camera 0,0,-1 : point camera 0,0,0
position light 0,0,5,-5 : point light 0,0,0,0
autocam on
REM ---------------------------------------------------------------------------------
REM TEMP MAIN
REM ---------------------------------------------------------------------------------
Disable escapekey : while escapekey()=0
for i = 1 to 5
Checkbtn3D(i)
next i
fastsync : endwhile
REM ---------------------------------------------------------------------------------
REM CLEAN UP MEDIA
REM ---------------------------------------------------------------------------------
for i = 1 to 5 : if object exist(i) = 1 : delete object i : endif : next i
for i = 1 to 5 : if image exist(i) = 1 : delete image i : endif : next i
end
REM ---------------------------------------------------------------------------------
REM FUNCTIONS
REM ---------------------------------------------------------------------------------
rem ------------------------------------------------------
rem Function By David Smith
rem ------------------------------------------------------
function Makebtn3D(objnum)
make object plain objnum,btn_siz_x(objnum),btn_siz_y(objnum)
position object objnum,0.0,0.0,0.0
lock object on objnum
ghost object on objnum
Placebtn3D(objnum)
endfunction
rem ------------------------------------------------------
rem Function By David Smith
rem ------------------------------------------------------
function Texture_3D_btn(objnum,btn_num_text$,txnum)
ink rgb(0,0,255),1
box 0,0,256,32
ink rgb(255,255,255),1
text 8,2,btn_num_text$
get image txnum,0,0,256,32,1
texture object objnum,txnum
endfunction
rem ------------------------------------------------------
rem Function by Thomas Christ
rem ------------------------------------------------------
Function Placebtn3D(objnum)
Width = Screen Width()/2
Height = Screen Height()/2
Z# = Screen Height()*0.83
Position Object objnum,(btn_x(objnum) - Width),(Height - btn_y(objnum)),Z#
EndFunction
rem ------------------------------------------------------
rem Function by David Smith
rem ------------------------------------------------------
Function Checkbtn3D(objnum)
btnxA = btn_x(objnum) - (btn_siz_x(objnum) / 2)
btnyA = btn_y(objnum) - (btn_siz_y(objnum) / 2)
btnxB = btn_x(objnum) + (btn_siz_x(objnum) / 2)
btnyB = btn_y(objnum) + (btn_siz_y(objnum) / 2)
If mousex()>btnxA and mousex()<btnxB and mousey()>btnyA and mousey()<btnyB and mouseclick()=0
scale object objnum,120,120,120
ghost object off objnum
else
ghost object on objnum
scale object objnum,100,100,100
endif
If mousex()>btnxA and mousex()<btnxB and mousey()>btnyA and mousey()<btnyB and mouseclick()=1
scale object objnum,110,110,110
ghost object off objnum
if objnum = 1
color backdrop rgb(150,0,0)
endif
if objnum = 2
color backdrop rgb(0,150,0)
endif
if objnum = 3
color backdrop rgb(150,150,0)
endif
if objnum = 4
color backdrop rgb(0,150,150)
endif
if objnum = 5
color backdrop rgb(0,0,0)
endif
endif
Endfunction
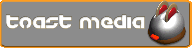