Updated the save function so it only saves one file instead of 2, and it now saves out editor variables so you can load later on and edit the ships.
Current bugs:
-when creating a new ship, hitting the Grid X Length for the first time after starting up the editor will cause it to go to zero, instead of staying at the default number. No idea why it does that.
-Using odd numbers for Grid sizes will result the x/z grid to have one extra grid point layer. You'll still be limited to your grid size and it won't effect the ship's center point. So just ignore it for now, lol.
Source Code:
RemStart *************************************
Blastwave Saga Ship Editor
Lee Mooney (C) 2007
Version 1.0
*************************************** RemEnd
Rem ******************************************
Rem Setup Display ****************************
Set Display Mode 1024,768,16 : Set Window On
Set Window Layout 3,1,0 : Set Window Title "BWS Ship Editor | V1.0"
Sync Rate 0 : Sync On : Sync Rate 60
Autocam Off : Backdrop On : Color Backdrop 0
Rem ******************************************
Rem Declare Types for Ship *******************
Type Ship_Stats `stats that deal with the entire ship
Name as String `name of ship
Object as Float `object number used for controlling and limb offsets
Speed as Float `ships max speed
Accel as Float `ships acceleration rate
Faction as Float `assosiated faction
AI as Float `level of AI in combat
EndType
Rem Declare Types for Limbs ******************
Type Limb_Stats `stats dealing with individual limbs
Name as String `name of limb
HP as Float `limbs hit points
SH as Float `limbs sheild points
Style as Float `limbs style
Output as Float `how much damage(if weapon), speed(if engine) or protection(if sheild) limb deals
Object as Float `object number used for this limb
Model as String `model name used (limbs directory)
Map as String `texture map used (maps directory)
X_Offset as Float `limbs offset in the X direction (ship orientation)
Y_Offset as Float `limbs offset in the Y direction (ship orientation)
Z_Offset as Float `limbs offset in the Z direction (ship orientation)
X_Angle as Float `limbs x angle offset (ships orientation)
Y_Angle as Float `limbs y angle offset (ships orientation)
Z_Angle as Float `limbs z angle offset (ships orientation)
Scale as Float `scale offset for the limb
NodeA_X as Float `node a's x offset (limb orientation)
NodeA_Y as Float `node a's y offset (limb orientation)
NodeA_Z as Float `node a's z offset (limb orientation)
NodeB_X as Float `node b's x offset (limb orientation)
NodeB_Y as Float `node b's y offset (limb orientation)
NodeB_Z as Float `node b's z offset (limb orientation)
EndType
Rem Declare Arrays with Types ****************
Dim Ship(1) as Ship_Stats : Ship(1).Name="default" `main ship array(only need one for editor)
Dim Limb(1,100) as Limb_Stats `ships limbs array
Limb_Amount as Float : Limb_Amount=0.0 `how many Limbs ship currently has
Rem ******************************************
Rem Declare Editor Variables *****************
Cursor_X# = 0 `position cursor x
Cursor_Y# = 0 `position cursor y
Cursor_Z# = 0 `position cursor z
Cursor_AX# = 0 `rotate cursor x
Cursor_AY# = 0 `rotate cursor y
Cursor_AZ# = 0 `rotate cursor z
Grid_X as FLoat = 10.0 `workspace x size
Grid_Y as Float = 10.0 `workspace y size
Grid_Z as Float = 10.0 `workspace z size
Camera_A# = 180 `camera angle
Zoom# = 10 `camera zoom range
Camera_S# = 1 `check if camera is on
Max_Objects# = 150 `how many objects can be used in the editor
Global Key_Input `globals for input
Global ReturnString$
Global S_value$
Rem ******************************************
Rem Declare Menu Variables *******************
Type Button_Stats `declare button type
Name as String `name of button function
State as Float `state of button 1/0
Xpos as Float `x position of button
Ypos as Float `y position of button
EndType
Rem Buttons Array ****************************
Dim Menu_Button(20) as Button_Stats `declare menu buttons array
Rem Set Menu Buttons Values ******************
For T = 1 to 20 : Menu_Button(T).State = 0.0 : Next T `set states to 0
Rem Set Button Stats *************************
Menu_Button(1).Name = " MENU" : Menu_Button(1).Xpos = 995.0 : Menu_Button(1).Ypos = 755.0
Menu_Button(2).Name = " NEW" : Menu_Button(2).Xpos = 995.0 : Menu_Button(2).Ypos = 739.0
Menu_Button(3).Name = " SAVE" : Menu_Button(3).Xpos = 995.0 : Menu_Button(3).Ypos = 723.0
Menu_Button(4).Name = " LOAD" : Menu_Button(4).Xpos = 995.0 : Menu_Button(4).Ypos = 707.0
Menu_Button(5).Name = " LIMB" : Menu_Button(5).Xpos = 995.0 : Menu_Button(5).Ypos = 691.0
Menu_Button(6).Name = " MAP" : Menu_Button(6).Xpos = 995.0 : Menu_Button(6).Ypos = 675.0
Menu_Button(7).Name = "STATS" : Menu_Button(7).Xpos = 995.0 : Menu_Button(7).Ypos = 659.0
Menu_Button(8).Name = "CLOSE" : Menu_Button(8).Xpos = 512.0 : Menu_Button(8).Ypos = 380.0
Menu_Button(9).Name = "X SIZE" : Menu_Button(9).Xpos = 512.0 : Menu_Button(9).Ypos = 308.0
Menu_Button(10).Name = "Y SIZE" : Menu_Button(10).Xpos = 512.0 : Menu_Button(10).Ypos = 326.0
Menu_Button(11).Name = "Z SIZE" : Menu_Button(11).Xpos = 512.0 : Menu_Button(11).Ypos = 344.0
Menu_Button(12).Name = "CREATE" : Menu_Button(12).Xpos = 512.0 : Menu_Button(12).Ypos = 362.0
Menu_Button(13).Name = "YES" : Menu_Button(13).Xpos = 462.0 : Menu_Button(13).Ypos = 380.0
Menu_Button(14).Name = "NO" : Menu_Button(14).Xpos = 562.0 : Menu_Button(14).Ypos = 380.0
Rem ******************************************
Rem Setup Editor Work Area *******************
Make Matrix 1,Grid_X,Grid_Z,Grid_X,Grid_Z `work area grid (can be adjusted in editor)
Position Matrix 1,-(Grid_X/2),0,-(Grid_Z/2) : Set Matrix 1,1,0,1,0,1,1,1
Rem Make Cursor Objects **********************
Make Object Cube 1,1 : Color Object 1,RGB(200,20,20)
Set Object Wireframe 1,1 : Set Object Ambient 1,100
Position Object 1,Cursor_X#,Cursor_Y#,Cursor_Z#
Rotate Object 1,Cursor_AX#,Cursor_AY#,Cursor_AZ#
Set Ambient Light 25 : Set Object Cull 1,0
Rem ******************************************
Rem Main Loop ********************************
Do
Rem ******************************************
Rem Print Variables for Testing **************
Set Cursor 0,0 `cursor position
INK RGB(255,255,255),RGB(0,0,0)
Print "MouseX: ";MouseX()
Print "MouseY: ";MouseY()
Print "Grid X: ";Grid_X
Print "Grid Y: ";Grid_Y
Print "Grid Z: ";Grid_Z
Print "Menu: ";Menu_Button(1).State
for x = 2 to 7 : Print "";Menu_Button(x).Name+": "+Str$(Menu_Button(x).State) : Next x
Rem ******************************************
Rem Gosubs ***********************************
Gosub HUD
Gosub Menu
Gosub Camera
Gosub Cursor_Control
Sync
Loop
Rem ******************************************
Rem Camera ***********************************
Camera:
Rem Camera Key Skip Check ********************
If Camera_Key <> Scancode() And Camera_S#=1
Rem Set Camera Angle *************************
If LeftKey()=1 Then Camera_A#=Wrapvalue(Camera_A#+90) `rotate camera clockwise
If RightKey()=1 Then Camera_A#=Wrapvalue(Camera_A#-90) `rotate camera counter clockwise
Rem Camera Key Skip **************************
Endif `end key spam
Camera_Key = Scancode() `get key for spaming
Rem Camera Zoom ******************************
If Upkey()=1 Then Inc Zoom#,-.5 : If Zoom#<1 Then Zoom#=1`zoom camera out
If Downkey()=1 Then Inc Zoom#,.5 `zoom camera in
Rem Set Camera to Follow Cursor **************
Set Camera to Follow Cursor_X#,Cursor_Y#,Cursor_Z#,Camera_A#,Zoom#,Cursor_Y#+(Zoom#/2),15,0
Point Camera Cursor_X#,Cursor_Y#,Cursor_Z# `point camera at cursor
Rem ******************************************
Rem Return to Main Code **********************
Return
Rem ******************************************
Rem Cursor Control ***************************
Cursor_Control:
Rem ******************************************
Rem Check Key Skip Check *********************
If Control_Key<>Scancode() And Camera_S#=1
Rem ******************************************
Rem Keyboard Movement Based On Camera Angle **
If Camera_A# = 0 `camera at 0 degrees
If Keystate(17)=1 Then Inc Cursor_Z#,1 `move cursor in the z direction(+)
If Keystate(31)=1 Then Inc Cursor_Z#,-1 `move cursor in the z direction(-)
If Keystate(30)=1 Then Inc Cursor_X#,-1 `move cursor in the x direction(-)
If Keystate(32)=1 Then Inc Cursor_X#,1 `move cursor in the x direction(+)
Endif
If Camera_A# = 90 `camera at 90 degrees
If Keystate(17)=1 Then Inc Cursor_X#,1 `move cursor in the x direction(+)
If Keystate(31)=1 Then Inc Cursor_X#,-1 `move cursor in the x direction(-)
If Keystate(32)=1 Then Inc Cursor_Z#,-1 `move cursor in the z direction(-)
If Keystate(30)=1 Then Inc Cursor_Z#,1 `move cursor in the z direction(+)
Endif
If Camera_A# = 180 `camera at 180 degrees
If Keystate(17)=1 Then Inc Cursor_Z#,-1 `move cursor in the z direction(-)
If Keystate(31)=1 Then Inc Cursor_Z#,1 `move cursor in the z direction(+)
If Keystate(30)=1 Then Inc Cursor_X#,1 `move cursor in the x direction(+)
If Keystate(32)=1 Then Inc Cursor_X#,-1 `move cursor in the x direction(-)
Endif
If Camera_A# = 270 `camera at 270 degrees
If Keystate(17)=1 Then Inc Cursor_X#,-1 `move cursor in the x direction(-)
If Keystate(31)=1 Then Inc Cursor_X#,1 `move cursor in the x direction(+)
If Keystate(32)=1 Then Inc Cursor_Z#,1 `move cursor in the z direction(+)
If Keystate(30)=1 Then Inc Cursor_Z#,-1 `move cursor in the z direction(-)
Endif
Rem ******************************************
Rem Move Cursor In Y Direction ***************
If Keystate(18)=1 Then Inc Cursor_Y#,1 `move cursor in y direction(+)
If Keystate(16)=1 Then Inc Cursor_Y#,-1 `move cursor in y direction(-)
Rem Control Key Skip ***********************
Endif `end key spam
Control_Key=Scancode() `get key for spaming
Rem ******************************************
Rem Check for out of bounds ******************
If Camera_S#=1
If Cursor_X#>(Grid_X/2) Then Cursor_X#=(Grid_X/2)
If Cursor_X#<-(Grid_X/2) Then Cursor_X#=-(Grid_X/2)
If Cursor_Y#>(Grid_Y/2) Then Cursor_Y#=(Grid_Y/2)
If Cursor_Y#<-(Grid_Y/2) Then Cursor_Y#=-(Grid_Y/2)
If Cursor_Z#>(Grid_Z/2) Then Cursor_Z#=(Grid_Z/2)
If Cursor_Z#<-(Grid_Z/2) Then Cursor_Z#=-(Grid_Z/2)
Endif
Rem ******************************************
Rem Update Cursor Position *******************
Position Object 1,Cursor_X#,Cursor_Y#,Cursor_Z#
Rotate Object 1,Cursor_AX#,Cursor_AY#,Cursor_AZ#
Rem ******************************************
Rem Return To Main Code **********************
Return
Rem ******************************************
Rem HUD **************************************
HUD:
Rem Grab Camera View String ******************
If Camera_A#=90 Then Camera$="LEFT"
If Camera_A#=0 Then Camera$="BACK"
If Camera_A#=180 Then Camera$="FRONT"
If Camera_A#=270 Then Camera$="RIGHT"
Rem Create Text Bar For Stats ****************
INK RGB(175,180,215),RGB(0,0,0) `set ink for HUD boxes
Box 0,752,1024,768 `bottom HUD box
Rem Print Cursor Stats ***********************
INK RGB(0,0,0),RGB(0,0,0) `set ink for text
Set Text Size 16 `set text size for HUD
Text 2,753,"X:"+STR$(Cursor_X#)+" Y:"+STR$(Cursor_Y#)+" Z:"+STR$(Cursor_Z#)+" AX:"+STR$(Cursor_AX#)+" AY:"+STR$(Cursor_AY#)+" AZ:"+STR$(Cursor_AZ#)+" View:"+Camera$ `print cursor position
Rem ******************************************
Rem Return to Main ***************************
Return
Rem ******************************************
Rem Menu Code ********************************
Menu:
Rem Check Mouse Spam *************************
If Mouse_Click<>1 `check and skip
Rem Setup Main Menu Check ********************
Select Menu_Button(1).State `check state
Rem Main Menu Closed *************************
Case 0.0 `if closed
INK RGB(0,0,0),RGB(0,0,0) : Set Text Size 16 `set text color / size
Center Text Menu_Button(1).Xpos,Menu_Button(1).Ypos,Menu_Button(1).Name `print button name
If Button_Hit(1)=1 Then Menu_Button(1).State=1.0 `check for menu button hit
EndCase
Rem Main Menu Open ***************************
Case 1.0 `if open
INK RGB(200,20,20),RGB(0,0,0) : Set Text Size 16 `set text color / size
Center Text Menu_Button(1).Xpos,Menu_Button(1).Ypos,Menu_Button(1).Name `print button name
If Button_Hit(1)=1 Then Menu_Button(1).State=0.0 `check for menu button hit
Rem Make and show menu buttons ***************
INK RGB(175,180,215),RGB(0,0,0) `set ink for Menu Box
BOX 960,655,1024,755 `make menu box
INK RGB(0,0,0),RGB(0,0,0) : Set Text Size 16 ` set text color and size
For A = 2 To 7 `print button and check for
Center Text Menu_Button(A).Xpos,Menu_Button(A).Ypos,Menu_Button(A).Name `print button name
If Button_Hit(A)=1 `check for button hits
Menu_Button(A).State=1.0 `open menu
Menu_Button(1).State=0.0 `close main menu
Endif
Next A
EndCase
EndSelect
Rem ******************************************
Rem New Menu *********************************
If Menu_Button(2).State=1.0 `check if opened
Menu_Button(1).State=0.0 `keep main menu closed
Rem Create Menu Box and Buttons **************
INK RGB(175,180,215),RGB(0,0,0) `set ink for Menu Box
BOX 462,300,562,400 `make menu box
INK RGB(0,0,0),RGB(0,0,0) `set ink for text
Rem Print Buttons ****************************
Center Text Menu_Button(8).Xpos,Menu_Button(8).Ypos,Menu_Button(8).Name
If Menu_Button(9).State=1.0 Then INK RGB(200,20,20),RGB(0,0,0)
Center Text Menu_Button(9).Xpos,Menu_Button(9).Ypos,Menu_Button(9).Name+":"+STR$(Grid_X)
INK RGB(0,0,0),RGB(0,0,0) `set ink for text
If Menu_Button(10).State=1.0 Then INK RGB(200,20,20),RGB(0,0,0)
Center Text Menu_Button(10).Xpos,Menu_Button(10).Ypos,Menu_Button(10).Name+":"+STR$(Grid_Y)
INK RGB(0,0,0),RGB(0,0,0) `set ink for text
If Menu_Button(11).State=1.0 Then INK RGB(200,20,20),RGB(0,0,0)
Center Text Menu_Button(11).Xpos,Menu_Button(11).Ypos,Menu_Button(11).Name+":"+STR$(Grid_Z)
INK RGB(0,0,0),RGB(0,0,0) `set ink for text
Center Text Menu_Button(12).Xpos,Menu_Button(12).Ypos,Menu_Button(12).Name
Rem Check for close button *******************
If Button_Hit(8)=1
For A = 9 to 11 : Menu_Button(A).State=0.0 : Next A `reset new buttons
Menu_Button(2).State=0.0 `close new menu
Endif
Rem Check for Grid Buttons *******************
If Button_Hit(9)=1 Then Menu_Button(9).State=1.0
If Button_Hit(10)=1 Then Menu_Button(10).State=1.0
If Button_Hit(11)=1 Then Menu_Button(11).State=1.0
Rem Check for Create Button ******************
If Button_Hit(12)=1
Rem Reset Menus ******************************
For A = 9 to 11 : Menu_Button(A).State=0.0 : Next A `reset new buttons
Menu_Button(2).State=0.0 `close new menu
Rem Create New Scene Using Variables *********
Delete Matrix 1 `delete current matrix
Make Matrix 1,Grid_X,Grid_Z,Grid_X,Grid_Z `work area grid
Position Matrix 1,-(Grid_X/2),0,-(Grid_Z/2) : Set Matrix 1,1,0,1,0,1,1,1
Cursor_X# = 0 `reset cursor pos/rot
Cursor_Y# = 0
Cursor_Z# = 0
Cursor_AX# = 0
Cursor_AY# = 0
Cursor_AZ# = 0
Camera_A# = 180 `reset camera angle/zoom
Zoom# = 10
Rem Delete Scene *****************************
For E = 2 to Max_Objects#
If Object Exist(E)=1 Then Delete Object E
Next E
Endif
Rem Check For XYZ buttons ********************
If Menu_Button(9).State=1.0 `x position
Camera_S#=0 `disable controls
Menu_Button(10).State=0.0 : Menu_Button(11).State=0.0 `keep other variables closed
Grid_X = VAL(Menu_Input(STR$(Grid_X))) `input x size
If ReturnKey()=1 Then Menu_Button(9).State=0.0 : Camera_S#=1
Endif
If Menu_Button(10).State=1.0 `y position
Camera_S#=0 `disable controls
Menu_Button(9).State=0.0 : Menu_Button(11).State=0.0 `keep other variables closed
Grid_Y = VAL(Menu_Input(STR$(Grid_Y))) `input x size
If ReturnKey()=1 Then Menu_Button(10).State=0.0 : Camera_S#=1
Endif
If Menu_Button(11).State=1.0 `z position
Camera_S#=0 `disable controls
Menu_Button(9).State=0.0 : Menu_Button(10).State=0.0 `keep other variables closed
Grid_Z = VAL(Menu_Input(STR$(Grid_Z))) `input x size
If ReturnKey()=1 Then Menu_Button(11).State=0.0 : Camera_S#=1
Endif
Rem End New Menu Code ************************
Endif
Rem ******************************************
Rem Save Menu Code ***************************
If Menu_Button(3).State=1.0 `check if open
Menu_Button(1).State=0.0 `keep main menu closed
Rem Check For save file **********************
INK RGB(175,180,215),RGB(0,0,0) `set ink for Menu Box
BOX 412,300,612,400 `make save box
Rem Print text and Buttons *******************
INK RGB(0,0,0),RGB(0,0,0)
Center Text 512,326,"Do you wish to save?"
Center Text Menu_Button(13).Xpos,Menu_Button(13).Ypos,Menu_Button(13).Name
Center Text Menu_Button(14).Xpos,Menu_Button(14).Ypos,Menu_Button(14).Name
Rem Save File ********************************
If Button_Hit(14)=1 `no button
Menu_Button(3).State=0.0 `close save window
Endif
If Button_Hit(13)=1 `yes button
Rem Save Ship File ***************************
If File Exist("ships\"+Ship(1).Name+".shp")=1 Then Delete File "ships\"+Ship(1).Name+".shp"
Open To Write 1,"ships\"+Ship(1).Name+".shp"
Write Float 1,Grix_X
Write Float 1,Grid_Y
Write Float 1,Grid_Z
Write String 1,Ship(1).Name
Write Float 1,Ship(1).Object
Write Float 1,Ship(1).Speed
Write Float 1,Ship(1).Accel
Write Float 1,Ship(1).Faction
Write Float 1,Ship(1).AI
Write Float 1,Limb_Amount
Rem Save Ship Limb Info **********************
If Limb_Amount>0.0 `check for limb exist
For L = 1 to Limb_Amount `write each limb in order
Write String 1,Limb(1,L).Name
Write Float 1,Limb(1,L).HP
Write Float 1,Limb(1,L).SH
Write Float 1,Limb(1,L).Style
Write Float 1,Limb(1,L).Output
Write Float 1,Limb(1,L).Object
Write String 1,Limb(1,L).Model
Write String 1,Limb(1,L).Map
Write Float 1,Limb(1,L).X_Offset
Write Float 1,Limb(1,L).Y_Offset
Write Float 1,Limb(1,L).Z_Offset
Write Float 1,Limb(1,L).X_Angle
Write Float 1,Limb(1,L).Y_Angle
Write Float 1,Limb(1,L).Z_Angle
Write Float 1,Limb(1,L).Scale
Write Float 1,Limb(1,L).NodeA_X
Write Float 1,Limb(1,L).NodeA_Y
Write Float 1,Limb(1,L).NodeA_Z
Write Float 1,Limb(1,L).NodeB_X
Write Float 1,Limb(1,L).NodeB_Y
Write Float 1,Limb(1,L).NodeB_Z
Next L
Endif
Close File 1
Rem Close save window ************************
Menu_Button(3).State=0.0
Endif
Rem End Save Menu Button *********************
Endif
Rem End Menu *********************************
Endif `menu skip for spaming
Mouse_Click=Mouseclick() `set mouseclick state
Rem ******************************************
Rem Return to main ***************************
Return
Rem ******************************************
Rem Button Hit Function **********************
Function Button_Hit(B) `check for button hit and change state
Returnvalue=0 `reset value
If MouseX()>Menu_Button(B).Xpos-(Text Width(Menu_Button(B).Name)/2)
If MouseX()<Menu_Button(B).Xpos+(Text Width(Menu_Button(B).Name)/2)
If MouseY()>Menu_Button(B).Ypos
If MouseY()<Menu_BUtton(B).Ypos+Text Height(Menu_BUtton(B).Name)
If Mouseclick()=1
ReturnValue=1
Endif
Endif
Endif
Endif
Endif
EndFunction Returnvalue
Rem ******************************************
Rem Model Exist Check ************************
Function Object_Check(first,last)
For C = first to last
If Object Exist(C)=0 Then ReturnObject=C Else ReturnObject=0
Next C
EndFunction ReturnObject
Rem ******************************************
Rem Input Function ***************************
Function Menu_Input(String_I$)
If Key_Input<>Scancode() `check for keyspam
Rem Check For Key and set value **************
Select Scancode()
Case 2 : S_value$="1" : EndCase
Case 3 : S_value$="2" : EndCase
Case 4 : S_value$="3" : EndCase
Case 5 : S_value$="4" : EndCase
Case 6 : S_value$="5" : EndCase
Case 7 : S_value$="6" : EndCase
Case 8 : S_value$="7" : EndCase
Case 9 : S_value$="8" : EndCase
Case 10 : S_value$="9" : EndCase
Case 11 : S_value$="0" : EndCase
Case 16 : S_value$="Q" : EndCase
Case 17 : S_value$="W" : EndCase
Case 18 : S_value$="E" : EndCase
Case 19 : S_value$="R" : EndCase
Case 20 : S_value$="T" : EndCase
Case 21 : S_value$="Y" : EndCase
Case 22 : S_value$="U" : EndCase
Case 23 : S_value$="I" : EndCase
Case 24 : S_value$="O" : EndCase
Case 25 : S_value$="P" : EndCase
Case 30 : S_value$="A" : EndCase
Case 31 : S_value$="S" : EndCase
Case 32 : S_value$="D" : EndCase
Case 33 : S_value$="F" : EndCase
Case 34 : S_value$="G" : EndCase
Case 35 : S_value$="H" : EndCase
Case 36 : S_value$="J" : EndCase
Case 37 : S_value$="K" : EndCase
Case 38 : S_value$="L" : EndCase
Case 43 : S_value$="Z" : EndCase
Case 38 : S_value$="X" : EndCase
Case 38 : S_value$="C" : EndCase
Case 38 : S_value$="V" : EndCase
Case 38 : S_value$="B" : EndCase
Case 38 : S_value$="N" : EndCase
Case 38 : S_value$="M" : EndCase
Case Default : S_value$="" : EndCase
EndSelect
Rem Create ReturnString **********************
ReturnString$=String_I$+S_value$
Rem Creat ReturnString w/ Space **************
If Spacekey()=1 Then ReturnString$=ReturnString$+" "
Rem Delete Character *************************
If Keystate(14)=1 `check for backspace
delete=LEN(ReturnString$)-1 : If delete<0 Then delete=0
ReturnString$=Left$(ReturnString$,delete)
Endif
Endif
Key_Input=Scancode() `set key_input variable
EndFunction ReturnString$
Started to work on some limb objects with textures and normal / spec maps. Anyone willing to donate some ships limbs would be much appreciated. I need at least a few types in order to test out my limb and map functions which I'm currently programing.
Will update later tonight.
Later, BWM
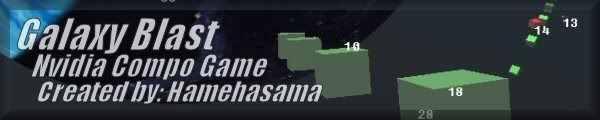
"I wish I was a wizard, becuase then I could wave my wand and perfect code would just appear."