Hi. I have made some color functions and I figured I'd post them here. You may or may not find then useful, but here they are. They're all very simple.
BrightenColor( color , brightness amount )
Will return the brightened version of a color. The COLOR parameter specifies the color, and the BRIGHTNESS AMOUNT specifies how much brightness you want to apply. Specifying a negative brightness amount will darken the color.
Function:
function BrightenColor(color as dword,amount as integer)
Red=RGBR(color)
Green=RGBG(color)
BLUE=RGBB(color)
Red=Red+amount
Green=Green+amount
Blue=Blue+amount
if Red>255 then Red=255
if Blue>255 then Blue=255
if Green>255 then Green=255
if Red<0 then Red=0
if Blue<0 then Blue=0
if Green<0 then Green=0
TEMP=rgb(Red,Green,Blue)
endfunction TEMP
Example:
rem Setup demo.
sync on
sync rate 60
set text font "Verdana"
set text size 12
rem Set the normal color
Color=rgb(255,155,0)
rem Brighten the normal color by 50.
Brighter=BrightenColor(Color,50)
rem Darken the normal color by 50.
Darker=BrightenColor(Color,-50)
do
rem Make the normal box.
ink color,0
box 0,0,100,100
rem Make the brighter box.
ink brighter,0
box 200,0,300,100
rem Make the darker box.
ink Darker,0
box 400,0,500,100
rem Label the boxes.
ink rgb(255,255,255),0
TEXT 0,150,"NORMAL COLOR"
TEXT 200,150,"BRIGHTER COLOR"
TEXT 400,150,"DARKER COLOR"
sync
loop
END
function BrightenColor(color as dword,amount as integer)
Red=RGBR(color)
Green=RGBG(color)
BLUE=RGBB(color)
Red=Red+amount
Green=Green+amount
Blue=Blue+amount
if Red>255 then Red=255
if Blue>255 then Blue=255
if Green>255 then Green=255
if Red<0 then Red=0
if Blue<0 then Blue=0
if Green<0 then Green=0
TEMP=rgb(Red,Green,Blue)
endfunction TEMP
ColorOpposite( color )
Will return the opposite of a color.
Function:
function ColorOpposite(color as dword)
RED=255-rgbr(color)
GREEN=255-rgbg(color)
BLUE=255-rgbb(color)
TEMP=rgb(RED,GREEN,BLUE )
endfunction TEMP
Example:
rem Setup demo.
sync on
sync rate 60
set text font "Verdana"
set text size 12
rem Set the normal color
Color=rgb(255,155,0)
rem Get the opposite of the original color.
Opposite=ColorOpposite( color )
do
rem Make the normal box.
ink color,0
box 0,0,100,100
rem Make the opposite box.
ink Opposite,0
box 200,0,300,100
rem Label the boxes.
ink rgb(255,255,255),0
TEXT 0,150,"NORMAL COLOR"
TEXT 200,150,"OPPOSITE COLOR"
sync
loop
END
function ColorOpposite(color as dword)
RED=255-rgbr(color)
GREEN=255-rgbg(color)
BLUE=255-rgbb(color)
TEMP=rgb(RED,GREEN,BLUE )
endfunction TEMP
Greyscale( color )
Returns the greyscale version of a color.
Function:
function GreyScale(color as dword)
RED=RGBR(color)
GREEN=RGBG(color)
BLUE=RGBB(color)
Amount=(RED+GREEN+BLUE)/3
TEMP=rgb(Amount,Amount,Amount)
endfunction TEMP
Example:
rem Setup demo.
sync on
sync rate 60
set text font "Verdana"
set text size 12
rem Set the normal color
Color=rgb(255,155,0)
rem Get the greyscale version of the color
Grey=Greyscale( color )
do
rem Make the normal box.
ink color,0
box 0,0,100,100
rem Make the opposite box.
ink Grey,0
box 200,0,300,100
rem Label the boxes.
ink rgb(255,255,255),0
TEXT 0,150,"NORMAL COLOR"
TEXT 200,150,"GREYSCALE COLOR"
sync
loop
END
function GreyScale(color as dword)
RED=RGBR(color)
GREEN=RGBG(color)
BLUE=RGBB(color)
Amount=(RED+GREEN+BLUE)/3
TEMP=rgb(Amount,Amount,Amount)
endfunction TEMP
-------------------------------------------------------------------
Here's a full example showing all of them in action:
sync on
sync rate 60
backdrop on
color backdrop 0
set text font "Verdana"
set text size 12
red=255
green=155
blue=26
Mode=1
Speed=2
do
if mode=1 then color=rgb(255,0,0)
if mode=2 then color=rgb(0,255,0)
if mode=3 then color=rgb(0,0,255)
ink color,0
box 0,0,50,50
if mousey()>=250 and mousey()<280 and mouseclick()=1 then Mode=1
if mousey()>=300 and mousey()<330 and mouseclick()=1 then Mode=2
if mousey()>=350 and mousey()<380 and mouseclick()=1 then Mode=3
if mode=1 then Red=Red+Speed*(upkey()-downkey())
if mode=2 then Green=Green+Speed*(upkey()-downkey())
if mode=3 then Blue=Blue+Speed*(upkey()-downkey())
if Red>255 then Red=255
if Blue>255 then Blue=255
if Green>255 then Green=255
if Red<0 then Red=0
if Blue<0 then Blue=0
if Green<0 then Green=0
Normal=rgb(red,green,blue)
Dark=BrightenColor(Normal,-50)
Light=BrightenColor(Normal,50)
ink rgb(255,255,255),0
TEXT 0,80,"LIGHTER"
TEXT 100,80,"NORMAL"
TEXT 200,80,"DARKER"
ink Light,0
box 0,100,100,200
ink Normal,0
box 100,100,200,200
ink Dark,0
box 200,100,300,200
c=-256
repeat
inc c
ink BrightenColor(Normal,c),0
box 100+c+255,Screen Height()-100,100+(c+255)+1,Screen Height()-75
ink ColorOpposite(BrightenColor(Normal,c)),0
box 100+c+255,Screen Height()-75,100+(c+255)+1,Screen Height()-50
ink GreyScale(BrightenColor(Normal,c)),0
box 100+c+255,Screen Height()-50,100+(c+255)+1,Screen Height()-25
until c=255
R$=""
G$=""
B$=""
if MODE=1 then R$=" *"
if MODE=2 then G$=" *"
if MODE=3 then B$=" *"
ink rgb(255,255,255),0
text 0,Screen height()-100,"DARK --> Light"
text 0,Screen height()-75,"Color Opposite"
text 0,Screen height()-50,"Greyscale"
line 0,screen height()-110,620,Screen Height()-110
line 620,Screen height()-110,620,screen height()
text 0,200,"Click on the color spelled out in order to change the amount of it. Use the UP/DOWN arrow keys to adjust the values."
ink rgb(255,0,0),0
TEXT 0,250,"RED: "+str$(RED)+R$
ink rgb(0,255,0),0
TEXT 0,300,"GREEN: "+str$(Green)+G$
ink rgb(0,0,255),0
TEXT 0,350,"BLUE: "+str$(BLUE)+B$
sync
loop
function BrightenColor(color as dword,amount as integer)
Red=RGBR(color)
Green=RGBG(color)
BLUE=RGBB(color)
Red=Red+amount
Green=Green+amount
Blue=Blue+amount
if Red>255 then Red=255
if Blue>255 then Blue=255
if Green>255 then Green=255
if Red<0 then Red=0
if Blue<0 then Blue=0
if Green<0 then Green=0
TEMP=rgb(Red,Green,Blue)
endfunction TEMP
function GreyScale(color as dword)
RED=RGBR(color)
GREEN=RGBG(color)
BLUE=RGBB(color)
Amount=(RED+GREEN+BLUE)/3
TEMP=rgb(Amount,Amount,Amount)
endfunction TEMP
function ColorOpposite(color as dword)
RED=255-rgbr(color)
GREEN=255-rgbg(color)
BLUE=255-rgbb(color)
TEMP=rgb(RED,GREEN,BLUE )
endfunction TEMP
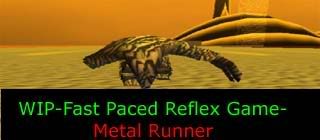