Here's my version. You can set a key (unique ID) so you can get different results for each key. For example, if you are checking mouse-clicks on sprites in one function and checking mouse clicks for objects in another function. It is also self-contained - no need to initialize. The function does differentiate between different mouse-clicks, so a left-click and then a simultaneous right-click will generate a two results (1st=left & 2nd=left+right).
The extra array sorting code is included to handle a large number of keys efficiently. Say for example; if someone had 1000 unique single-mouse-click checks for various reasons, the sorted array handles that large quantity efficiently.
For simplicity, you can ignore the code and just call the function:
ReturnValue = Single_MouseClick(Key as Integer)
`============================================================================================================================
`=SINGLE MOUSE-CLICK FUNCTIONS===============================================================================================
`============================================================================================================================
`intKey is a unique key identifier for use by different function calls. This way, the results stored by
`one function call are not used in the next function call. All funcion calls receive their own unique results.
Function Single_MouseClick(intKey as Integer)
`Initialization
Initialize_Array_MouseClicks()
`Declarations
Local intIndex as Integer = 0
Local intRV as Integer = 0
`Retireve the index (if one exists) for the provided Key
intIndex = MouseClicks_Find_Entity(intKey)
`Check to see if the Key was found. If not, then create a new key.
if intIndex<0
MouseClicks_Add_EntityID(intKey)
Endif
`Check to see if the previous mouse click matches the current mouseclick.
If MouseClicks(intIndex).ClickFlag = MouseClick()
`If there is a match, then return a 0 result
intRV=0
Else
`The last mouseclick and the current mouseclick are different, so a change is noted
`and the click value is returned.
intRV=MouseClick()
`Store the current Click results.
MouseClicks(intIndex).ClickFlag = intRV
Endif
Endfunction intRV
Type MouseClicks_Type
EntityID as Integer
ClickFlag as Integer
EndType
`Note: These functions are only intended as sub-functions to support the Single_MouseClick() function and should not be used/called
`externally.
Function Initialize_Array_MouseClicks()
`Defensive programming to prevent this function from being called more than once.
Dim InitArrayMouseClicks(0)
If InitArrayMouseClicks(0) Then ExitFunction
InitArrayMouseClicks(0)=1 : `Set the InitArrayMouseClicks flag to 1 to prevent the function from being called again.
`Declarations
Dim MouseClicks(999) as MouseClicks_Type
Dim MouseClicksTop(0) as Integer
MouseClicksTop(0) = -1
EndFunction
`MouseClicks_Find_Entity(EntityID as Integer):Return Integer
`Binary searches through a sorted array for the specified EntityID.
`The return integer EntityID is the index of the array element for which the EntityID was found.
`If there are multiples of the same EntityID within the array, any one of the indexes may be returned.
`If the EntityID is not found, a -1 is returned.
Function MouseClicks_Find_Entity(EntityID as Integer)
`Initialize the array functions
Initialize_Array_MouseClicks()
`Store the Top of the array in a local variable for easier reading/typing.
Top=MouseClicksTop(0)
`Calculate the half-way point in the array.
Half=(Top+1)/2
`If the array has no elements yet, exit the function and return a "false" result
If Top<0 Then ExitFunction -1
`Quick Check 1 to see if the search value is less than the lowest array value. If so, then the search
`value cannot exist within the array, so return a "false" result.
If EntityID<MouseClicks(0).EntityID Then ExitFunction -1
`Quick Check 2 to see if the search value is the first array value. Return a 0 (the first element index) if it is.
If EntityID=MouseClicks(0).EntityID Then ExitFunction 0
`Quick Check 3 to see if the search value is the last array value. Return the last element index if it is.
If EntityID=MouseClicks(Top).EntityID Then ExitFunction Top
`Quick Check 4 to see if the search value is greater than the largest array value. If so, then the search
`value cannot exist within the array, so return a "false" result.
If EntityID>MouseClicks(Top).EntityID Then ExitFunction -1
`Set the BSearch variable to half the array. The starting point of the search is in the middle of the array and searches up
`or down based on if the search value is greater than or less than the current array index element value.
BSearch=Half
`Begin the search loop
For i = 0 to 16:`Note, 15 is 2 Bytes worth of searching, or 2^15. 16 is used as overkill to ensure a complete search.
`Defensive programming. Ensure the search location cannot be a value that is not valid or makes no sense (i.e. the 1st and last elements were already checked).
If BSearch<1 then BSearch=1
If BSearch>Top then BSearch=Top
`Check to see if the current element contains the search value. If it does, return the array index for the element in which the value was found.
If EntityID=MouseClicks(BSearch).EntityID Then ExitFunction BSearch
`Reduce our half search by half again since we will know which half of the current search range the element has to be in (higher or lower)
Half=(Half+1)/2
`Defensive programming to ensure the half value never goes below 1 and causes problem.
If Half<1 then Half=1
`Calculate where our new search location will be, either half way up, or half way down.
BSearch=BSearch+Half*(((EntityID=>MouseClicks(BSearch).EntityID)-(EntityID<MouseClicks(BSearch).EntityID)))
Next i
`The value was not found durning the search. Return a "false" result.
EndFunction -1
`MouseClicks_Add_EntityID(EntityID As Integer):Return Integer
`Performs a binary search for an index location in which to insert a new array element
`for the specified EntityID in sort order of ascending EntityID's. The array element is added
`and the EntityID is recorded the new array element.
`The return value is the index (array element) where the new EntityID was placed.
Function MouseClicks_Add_EntityID(EntityID As Integer)
`Find the index location where to insert the new value
Index=MouseClicks_Find_EntityID_Place(EntityID)
`Insert a new element into the array
MouseClicks_Insert_Element(Index)
`Store the value in the new array position
MouseClicks(Index).EntityID=EntityID
`Return the new index location
EndFunction Index
`MouseClicks_Insert_Element(Index as Integer)
`Increases the array by adding in an element at the specified index.
`If the Index is larger than the array size, the new element is added at
`the end of the array. Otherwise, the new element is inserted the specified
`index.
Function MouseClicks_Insert_Element(Index as Integer)
`Initialization
Initialize_Array_MouseClicks()
`Increase the array Top by one.
MouseClicksTop(0)=MouseClicksTop(0)+1
`Check to see if the array Top has exceeded the size of the array
While MouseClicksTop(0)=>Array Count(MouseClicks())
`If yes, then increase the size of the array
Array Insert At Bottom MouseClicks()
`I converted this to a While loop, just to cover all possibilities.
EndWhile
`Defensive code. The index provided was not valid. This should never happen, but is included to be safe.
If Index=>MouseClicksTop(0) Then ExitFunction
`Copy all the elements from the new index location to the top of the array to next highest array elements.
`Believe it or not, this is faster than actually inserting a new array element.
For i =MouseClicksTop(0)-1 To Index Step -1
MouseClicks(i+1)=MouseClicks(i)
Next i
EndFunction
`MouseClicks_Find_EntityID_Place(EntityID as Integer):Return Integer
`This function is similar to the MouseClicks_Find_EntityID() function in that it
`searches for a EntityID within a sorted array. However, if the EntityID is
`not found in this function, an index EntityID is returned where the search EntityID
`"fits" within the sorted array. For example: The array - 1,6,8,9 and the EntityID
`is 7, the return element will be 2 since 7 falls between elements 1 and 2 (6 & 9).
`If the EntityID is lower than any EntityID within the array, a 0 is returned.
`If the EntityID is larger than any EntityID within the array, Array Count()+1 is returned.
Function MouseClicks_Find_EntityID_Place(EntityID as Integer)
`Initialize the array functions
Initialize_Array_MouseClicks()
`Store the Top of the array in a local variable for easier reading/typing.
Top=MouseClicksTop(0)
`Calculate the half-way point in the array.
Half=(Top+1)/2
`If the array has no elements yet, exit the function and return a 0 (the first element)
If Top<0 Then ExitFunction 0
`Quick Check 1: If the search value is less than the first element, return a 0 (the first element)
If EntityID<=MouseClicks(0).EntityID Then ExitFunction 0
`Quick Check 2: If the search value is more than the last element, return the Array size + 1.
If EntityID=>MouseClicks(Top).EntityID Then ExitFunction Top+1
`Set the BSearch variable to half the array. The starting point of the search is in the middle of the array and searches up
`or down based on if the search value is greater than or less than the current array index element value.
BSearch=Half
`Begin the search loop
For i = 0 to 16:`Note, 15 is 2 Bytes worth of searching, or 2^15 elements.
`Defensive programming. Ensure the search location cannot be a value that is not valid or makes no sense (i.e. the 1st and last elements were already checked).
If BSearch<1 then BSearch=1
If BSearch>Top then BSearch=Top
`Check to see if the current element contains the search value. If it does, return the array index for the element in which the value was found.
If EntityID=MouseClicks(BSearch).EntityID Then ExitFunction BSearch
`Check to see if the search value is between the current element and the next highest element.
`If it is, return the array index for the element in which the value was found. This is the "Insert" location.
If EntityID>MouseClicks(BSearch-1).EntityID And EntityID<=MouseClicks(BSearch).EntityID then ExitFunction BSearch
`Reduce our half search by half again since we will know which half of the current search range the element has to be in (higher or lower)
Half=(Half+1)/2
`Defensive programming to ensure the half value never goes below 1 and causes problem.
If Half<1 then Half=1
`Calculate where our new search location will be, either half way up, or half way down.
BSearch=BSearch+Half*(((EntityID=>MouseClicks(BSearch).EntityID)-(EntityID<MouseClicks(BSearch).EntityID)))
Next i
`The code should never execute the following lines since there is no logical way to arrive at this point since all other conditions have been
`checked for. However, just in case, this safety catch will return an index location at the array top + 1.
Top=Top+1
EndFunction Top
`MouseClicks_Delete_Element(Index as Integer)
`This function will delete an array element at the specified Index.
`If the index is not valid, the function will fail without exiting the program.
Function MouseClicks_Delete_Element(Index as Integer)
`Initialization
Initialize_Array_MouseClicks()
`Defensive programming to ensure the index provided is valid. If not, just exit the function.
If Index<0 or Index>MouseClicksTop(0) Then ExitFunction
`Reduce the array Top by one.
MouseClicksTop(0)=MouseClicksTop(0)-1
`Copy all the elements from the index to the array top down to the next lowest element.
`The element at the index location is copied over and therefore "deleted" by default.
For i = Index to MouseClicksTop(0)
MouseClicks(i)=MouseClicks(i+1)
Next i
EndFunction
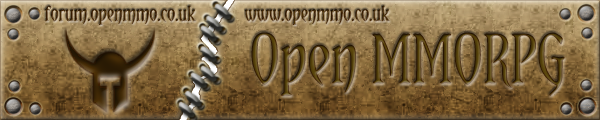
Open MMORPG: It's your game!