Hey all,
I have recently come across some good shader tutorials, but they are written for XNA, not DGDK.
I was wondering if anyone knew any XNA and would be able to help me solve a problem, for a specular lighting shader.
The following code is the XNA command to pass the appropriate variable to the shader:
Vector3 cameraLocation = distance * new Vector3((float)Math.Sin(angle), 0, (float)Math.Cos(angle));
Vector3 cameraTarget = new Vector3(0, 0, 0);
viewVector = Vector3.Transform(cameraTarget - cameraLocation, Matrix.CreateRotationY(0));
viewVector.Normalize();
view = Matrix.CreateLookAt(cameraLocation, cameraTarget, new Vector3(0, 1, 0));
effect.Parameters["ViewVector"].SetValue(viewVector);
I understand most of it, what I just don't know is what the ViewVector should actually be!
The shader runs at the moment, but without the desired specular effect.
Thanks all!
EDIT:
Here is the shader code, I just realised it may help.
matrix WVP:WorldViewProjection;
matrix World:World;
matrix WorldInverseTranspose;
float4 AmbientColor = {1.0f, 1.0f, 1.0f, 1.0f};
float AmbientIntensity = 0.7f;
float3 DiffuseLightPosition = {0.0f, 50.0f, -10.0f};
float4 DiffuseColor = {1.0f, 1.0f, 1.0f, 1.0f};
float DiffuseIntensity = 0.7f;
float SpecularLevel = 200.0f;
float4 SpecularColor = {1.0f, 1.0f, 1.0f, 1.0f};
float SpecularIntensity = 0.7f;
float3 ViewVector = {1.0f, 1.0f, 1.0f};
struct VS_Input
{
float4 Position : POSITION0;
float4 Normal : NORMAL;
};
struct VS_Output
{
float4 Position : POSITION0;
float4 Color : COLOR0;
float4 Normal : TEXCOORD0;
};
VS_Output VS(VS_Input input)
{
VS_Output output;
output.Position = mul(input.Position,WVP);
float4 normal = mul(input.Normal, WorldInverseTranspose);
float lightIntensity = dot(normal, DiffuseLightPosition);
output.Color = saturate(DiffuseColor * DiffuseIntensity * lightIntensity);
output.Normal = normal;
return output;
}
float4 PS(VS_Output input) : COLOR
{
float3 light = normalize(DiffuseLightPosition);
float3 normal = normalize(input.Normal);
float3 r = normalize(2 * dot(light, normal) * normal - light);
float3 v = normalize(mul(normalize(ViewVector), World));
float dotProduct = dot(r, v);
float4 specular = SpecularIntensity * SpecularColor * max(pow(dotProduct, SpecularLevel), 0) * length(input.Color);
return saturate(input.Color + AmbientColor * AmbientIntensity + specular);
}
technique Lighting
{
pass Pass1
{
VertexShader = compile vs_2_0 VS();
PixelShader = compile ps_2_0 PS();
}
}
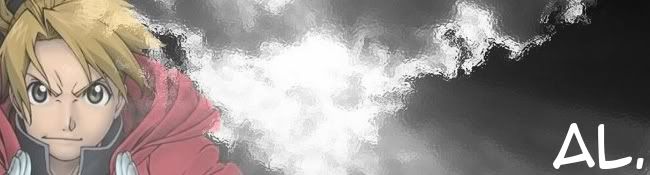