I'm implementing Box2D into
S3GE's Multi-App User Interface.
1. Download the Box2D zip.
2. Build the library in Release mode to generate box2d.lib. If you build in Debug mode you may get linker errors looking for missing debug libs when you build your project.
3. After successfully building the lib, You add the box2d.lib and box2d.h to your Project:
Project Properties -> Linker -> Input -> Additional Dependencies -> box2d.lib
Project Properties -> C/C++ -> General -> Additional Include Directories -> {
Where you placed the Box2D Include and Source Folders & Files}
4. If you get this far, then check out the
Box2D Manual for the example code to continue on to next steps.
5. Build a World Object. This is the first object you need to create and its basically a bounding box in which the simulation is managed. The size isn't critical, but a better fit will improve performance. It is better to make the box too big than to make it too small.
Box2D uses meters for units of scale, thus you will need to do some math conversions for equivalent pixel scale for DGDK. 1 meter = ~ 30 pixels.
I define some macros to ease calculations
//box2d constants
#define b2PixelRatio 30.0f
#define b2PixelToMeter(n) ((n)/b2PixelRatio)
#define b2MeterToPixel(n) ((n)*b2PixelRatio)
#define b2PixelToHalfMeter(n) (((n)/b2PixelRatio)/2)
#define b2HalfMeterToPixel(n) (((n)*b2PixelRatio)*2)
Creating a World Object. You can follow the example code in the Box2D manual. I only list code snippets that require math conversions.
worldAABB.lowerBound.Set(screenstartx,screenstarty);
worldAABB.upperBound.Set(screenwidth/b2PixelRatio, screenheight/b2PixelRatio);
6. Build a Body Objects. Bodies are physics objects and they are created in a very specific order:
i. Define a body with a position, damping, etc.
ii. Use the world object to create the body.
iii. Define shapes with geometry, friction, density, etc.
iv. Create shapes on the body.
v. Optionally adjust the body's mass to match the attached shapes.
For simple Rectangles Box2D creates them using half-width, half-height.
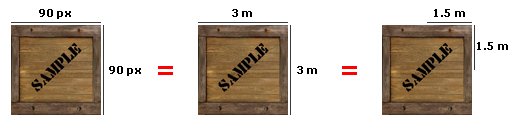
.
These measurements require you to offset the sprite/image position to be displayed to match DGDK position coords.
Creating a Body. You can follow the example code in the Box2D manual. I only list code snippets that require math conversions.
bodyDef.position.Set((rectstartx + (width/2.0f)) /b2PixelRatio, (rectstarty + (rectheight/2.0f)) /b2PixelRatio);
shapeDef.SetAsBox((rectwidth/b2PixelRatio)/2.0f, (rectheight/b2PixelRatio)/2.0f);
7. DGDK Sprites. As stated before you have to do some math conversions to display a Sprite equivalent to the physics sim. This requires you to offset the sprite prior to display. I offset them upon creation.
Offsetting DGDK Sprites
dbOffsetSprite(dbSpriteID, dbSpriteWidth(dbSpriteID)/2,dbSpriteHeight(dbSpriteID)/2);
Updating Objects. When updating each Body, perform your conversion and then display.
float32 angle = body->GetAngle();
b2Vec2 position = body->GetPosition();
position.x *= b2PixelRatio;
position.y *= b2PixelRatio;
angle *= (180.0 / 3.141592653589793238);
dbPasteSprite(dbSpriteID, position.x , position.y);
dbRotateSprite(dbSpriteID, angle);
I hope you find this helpful. Good Luck.