Quote: "but i can't find a way to replace my 'goto' commands"
Losing GOTO commands is a great idea. I view using GOTOs as a bad programming practice; if you structure your code properly, they are almost never needed. That said, there are some on here that think they are okay. To each his own.
Anyway, to eliminate the need for them, you could simply use GOSUB instead and make subroutines out of New_Game, HighScores, Difficulty, etc.
Quote: "Also with the AI, i had attempted one that only moves once the ball is towards the edge of the paddle, but thtis almost always resulted in a bad collision with the ball. Would you have any suggestions of how i could ammend this issue?"
I made a few quick changes to the code to give you an AI that is at least beatable. Check it out:
Rem Project: Dark Basic Pro Project
Rem Created: Sunday, January 30, 2011
Rem ***** Main Source File *****
Rem Project: Dark Basic Pro Project
Rem Created: Saturday, January 29, 2011
Rem ***** Main Source File *****
sync on : sync rate 60
hide mouse : randomize timer()
rem Screen coordinates
ScrWid = screen width() : ScrHgt = screen height()
rem PLAYER
PX1=15 : PY1=10 : PX2=25 : PY2=90
PR1=0 : PG1=255 : PB1=0
rem ENEMY
EX1 = ScrWid - 25 : EX2 = EX1 + 10
EY1 = ScrHgt / 2 : EY2 = EY1 + 80
ER1=255 : EG1=0 : EB1=0
rem BALL
BallX=ScrWid / 2 : BallY=ScrHgt / 2
BallSize = 10
SpeedX = 5 : SpeedY = 3
` randomly choose a direction
if rnd(100) > 50 then SpeedX = SpeedX * -1 : ` 50% chance it will go left or right
if rnd(100) < 50 then SpeedY = SpeedY * -1 : ` 50% chance it will be moving up or down
Border = 25
background = 1 : BallImg = 2 : PPad = 3 : Epad = 4
PScore = 0 : EScore = 0
gosub GetImages
Do
` instead of clearing the screen, we paste the image over it
paste image background,0,0
rem DRAW PLAYER PADDLE
sprite PPad,PX1,PY1,PPad : ` place the player's paddle based upon the variables
PY1=mousey()-40
PY2=mousey()+40
rem DRAW ENEMY PADDLE
sprite EPAd,EX1,Ey1,EPad : ` place enemy's paddle
` move enemy paddle
if Bally < EY1
if EY1 > 8 then dec EY1,6
else
if BallY > (EY1 + 80) and EY1 < (ScrHgt - 88) then inc EY1,6
ENDIF
rem BALL MOVEMENT
BallX=BallX+SpeedX
BallY=BallY+SpeedY
if BallY > ScrHgt - Border
BallY = ScrHgt - Border
SpeedY = SpeedY * -1
else
if BallY < Border
BallY = Border
SpeedY = SpeedY*-1
endif
endif
` place the ball sprite based upon the variables
sprite BallImg,BallX,BallY,BallImg
` check to see if there was a collision between the ball and the
` player's paddle or the enemy's paddle - if so, reverse the X direction
if sprite collision(BallImg,PPad) = 1 or sprite collision(BallImg,EPad) = 1
SpeedX=SpeedX*-1
endif
` did the ball go out of bounds? If so, add a point to the other player.
` Restart is a subroutine that prints out who won that round and what the
` current scores are
if BallX < PX1 then inc EScore : Winner = 2 : gosub Restart
if BallX > EX2 then inc PScore : Winner = 1 : gosub Restart
sync
Loop
end
GetImages:
` make a separate bitmap that we can use to create images
` by default, it becomes the current bitmap
` make it as large as the screen, so that we can make
` a background image, as well as the others needed
create bitmap 1,ScrWid,ScrHgt
// main background image
ink rgb(255,255,0),0
line 2,2,ScrWid - 2,2
line 2,2,2,ScrHgt - 2
line ScrWid - 2,2,ScrWid - 2,ScrHgt - 2
line 2,ScrHgt - 2,ScrWid - 2,ScrHgt - 2
line ScrWid / 2,2,ScrWid / 2,ScrHgt - 2
` grab the entire screen as an image
get image background,0,0,ScrWid - 1,ScrHgt - 1
cls : ` this clears bitmap 1
// ball image
ink rgb(255,255,0),0
Circle BallSize ,BallSize ,BallSize
get image BallImg,0,0,(BallSize * 2) + 1,(BallSize * 2) + 1
` make a sprite using this image
sprite BallImg,BallX,BallY,BallImg
cls // player paddle
ink rgb(PR1,PG1,PB1),0
box PX1,PY1,PX2,PY2
get image PPad,PX1,PY1,PX2,PY2
`make the player paddle sprite using this image
Sprite PPad,PX1,PY1,PPad
cls // DRAW ENEMY PADDLE
ink rgb(255,0,0),0
box EX1,EY1,EX2,EY2
get image EPad,EX1,EY1,EX2,EY2
` make the enemy paddle sprite using this image
sprite EPad,EX1,EY1,EPad
` bitmap 0 is the one normally used for all drawing
set current bitmap 0
` we don't need bitmap 1 any more, so we'll delete it
delete bitmap 1
return
Restart:
` make sure there are no mouse buttons pressed
repeat
paste image background,0,0
sync
UNTIL mouseclick() = 0
` pre-calculate the main position for our texts
xpos = ScrWid / 2 : ypos = ScrHgt / 2
if Winner = 1
ink rgb(PR1,PG1,PB1),0 : // player
else
ink rgb(255,0,0),0 : // enemy
ENDIF
` keep repeating this until a mouse button is clicked
repeat
paste image background,0,0
center text xpos,ypos,"Player " + str$(Winner) + " scored a point!"
center text xpos,ypos + 30,"Player: " + str$(PScore) + " Enemy: " + str$(EScore)
center text xpos,ypos + 60,"Click the mouse to play again."
sync
UNTIL mouseclick() > 0
` reset the ball coordinates so that it is at the center of the screen
BallX = ScrWid / 2 : BallY = ScrHgt / 2 : EY1 = BallY
` reset the ball X and Y speeds
SpeedX = 5 : SpeedY = 3
` randomly pick the direction of the ball
if rnd(100) > 50 then SpeedX = SpeedX * -1
if rnd(100) < 50 then SpeedY = SpeedY * -1
return
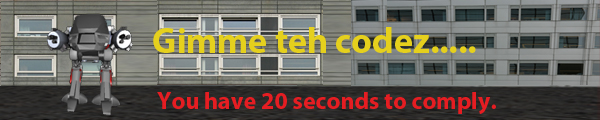