Yea I have noticed that, it has to do with the flood fill. It looks as though when suddenly changing the direction of movement, the lines don't match up in the first iteration and so the flood fill 'escapes' out. I'm looking to correct it. Here's an updated version, the flash hasn't been corrected in it yet, this is more of an updated work in progress.
Version 2:
REM =======================================================================
REM Author: Phaelax
REM Title: Amoeba
REM
REM Use up/down arrow keys or the mouse left/right buttons to move the
REM amoeba towards/away from the mouse cursor.
REM =======================================================================
type _segPt
x as float
y as float
alpha as float
endtype
segments = 40
radius = 100
angleStep# = 360.0 / segments
waveStrength# = 4.0
dim points(segments) as _segPt
randomize timer()
for i = 1 to segments
points(i).alpha = rnd(360)
next i
centerX = screen width() / 2
centerY = screen height() / 2
load sound "c:\slither2.wav", 1
sync on
sync rate 60
repeat
cls
rem amoeba will move towards the mouse cursor
mx = mousex()
my = mousey()
rem the radii of the ellipse is dependent upon how fast it moves
xRadius# = spd#*5
yRadius# = xRadius#/2
for i = 1 to segments
angle# = i*angleStep#
if i mod 2 = 0
points(i).alpha = wrapvalue(points(i).alpha+3)
alpha# = sin(points(i).alpha)*waveStrength#
endif
sina# = sin(angle#)
cosa# = cos(angle#)
x = sina#*(radius+xRadius#) + sina#*alpha#
y = cosa#*(radius-yRadius#) + cosa#*alpha#
x1 = x*sin(a#) - y*cos(a#)
y1 = y*sin(a#) + x*cos(a#)
x3 = centerX + x1/3
y3 = centerY + y1/3
inc x1, centerX
inc y1, centerY
points(i).x = x1
points(i).y = y1
rem get next point around ellipse to connect this line segment
if i < segments : j = i+1 : else : j = 1 : endif
`if i > 1 : j = i-1 : else : j = segments : endif
x = sin(j*angleStep#)*(radius+xRadius#)
y = cos(j*angleStep#)*(radius-yRadius#)
x2 = x*sin(a#) - y*cos(a#)
y2 = y*sin(a#) + x*cos(a#)
x4 = centerX + x2/3
y4 = centerY + y2/3
inc x2, centerX
inc y2, centerY
x2 = points(j).x
y2 = points(j).y
rem draw the shape
`ink rgb(75,130,46), 0
`line x1, y1, x2, y2
`ink 0xFFDAFF69, 0
`line x3, y3, x4, y4
`wuline(x1, y1, x2, y2)
next i
ink rgb(75,130,46), 0
for i = 1 to segments
j = i+1
if j > segments then j = 1
line points(i).x, points(i).y, points(j).x, points(j).y
`if i mod 2 = 0
r = sin(wrapvalue((i/(segments+0.0))*360 + ta))*4
a2# = atanfull(points(i).x-centerX, points(i).y-centerY)
line points(i).x, points(i).y, points(i).x+sin(a2#)*16+r, points(i).y+cos(a2#)*16+r
`endif
next i
ta = wrapvalue(ta+5)
rem fill in the shape (thx IanM!)
`rgb(126,191,55)
fill centerX+(radius+xRadius#)/2, centerY, rgb(75,130,46)
`fill points(1).x+6, points(1).y, rgb(75,130,246)
`fill centerX, centerY, 0xFFDAFF69
ink rgb(255,0,0), 0
`box points(1).x+6, points(1).y, points(1).x+2, points(1).y+2
rem controls; only move amoeba in small incremental jumps
if spd# = 0
if upkey() = 1 or mouseclick() = 1
sFlag = 1
a# = atanfull(mx-centerX, my-centerY)
play sound 1
endif
if downkey() = 1 or mouseclick() = 2
sFlag = 1
a# = atanfull(centerX-mx, centerY-my)
endif
endif
rem allows the amoeba appear to stretch out
if sFlag > 0
inc spd#, 2
centerX = centerX + sin(a#)*spd#
centerY = centerY + cos(a#)*spd#
if spd# >= 10 then sFlag = 0
endif
rem shrinks amoeba back into circle
if spd# > 0 then spd# = spd# - 0.5
set cursor 0,0
print "Speed: ", spd#
print screen fps()
sync
until spacekey()
end
REM Xiaolin Wu's line algorithm
function wuline(x1,y1,x2,y2)
local dx as float
local dy as float
local xend as float
local yend as float
local xgap as float
local ygap as float
local xpxl1 as float
local ypxl1 as float
local xpxl2 as float
local ypxl2 as float
local intery as float
local interx as float
dx = x2-x1
dy = y2-y1
if abs(dx) > abs(dy)
rem handle horizontal lines
if x2 < x1
ax = x1
x1 = x2
x2 = ax
ay = y1
y1 = y2
y2 = ay
endif
gradient# = dy / dx
rem handle first endpoint
xend = ceil(x1)
yend = y1 + gradient# * (xend-x1)
xgap = 1.0 - fract#(x1 + 0.5)
xpxl1 = xend : `used in main loop
ypxl1 = int(yend)
f# = 1-fract#(yend)*xgap : `brightness
c = 255*f#
ink rgb(c,c,c),0
dot xpxl1, ypxl1
rem brightness: fract#(yend)*xgap
f# = fract#(yend)*xgap : `brightness
c = 255*f#
ink rgb(c,c,c),0
dot xpxl1, ypxl1+1
intery = yend + gradient# : `first y-intersection for main loop
rem handle second endpoint
xend = ceil(x2)
yend = y2 + gradient# * (xend-x2)
xgap = 1.0 - fract#(x2 + 0.5)
xpxl2 = xend : `used in main loop
ypxl2 = int(yend)
f# = 1-fract#(yend)*xgap : `brightness
c = 255*f#
ink rgb(c,c,c),0
dot xpxl2, ypxl2
f# = fract#(yend)*xgap : `brightness
c = 255*f#
ink rgb(c,c,c),0
dot xpxl2, ypxl2+1
rem main loop
a = xpxl1+1
b = xpxl2-1
for x = a to b
f# = 1-fract#(intery) : `brightness
c = 255*f#
ink rgb(c,c,c),0
dot x, intery
f# = fract#(intery) : `brightness
c = 255*f#
ink rgb(c,c,c),0
dot x, intery+1
intery = intery + gradient#
next x
else
rem handle vertical lines
if y2 < y1
ax = x1
x1 = x2
x2 = ax
ay = y1
y1 = y2
y2 = ay
endif
gradient# = dx / dy
ink rgb(255,0,0),0
rem handle first endpoint
yend = ceil(y1)
xend = x1 + gradient# * (yend-y1)
ygap = 1.0 - fract#(y1 + 0.5)
xpxl1 = int(xend)
ypxl1 = yend
f# = 1-fract#(xend)*ygap : `brightness
c = 255*f#
ink rgb(c,c,c),0
dot xpxl1, ypxl1
f# = fract#(xend)*ygap : `brightness
c = 255*f#
ink rgb(c,c,c),0
dot xpxl1, ypxl1+1
interx = xend + gradient# : `first y-intersection for main loop
rem handle second endpoint
yend = ceil(y2)
xend = x2 + gradient# * (yend-y2)
ygap = fract#(y2 + 0.5)
xpxl2 = int(xend)
ypxl2 = yend
f# = 1-fract#(xend)*ygap : `brightness
c = 255*f#
ink rgb(c,c,c),0
dot xpxl2, ypxl2
f# = fract#(xend)*ygap : `brightness
c = 255*f#
ink rgb(c,c,c),0
dot xpxl2, ypxl2+1
rem main loop
a = ypxl1+1
b = ypxl2-1
for y = a to b
f# = 1-fract#(interx) : `brightness
c = 255*f#
ink rgb(c,c,c),0
dot interx, y
f# = fract#(interx) : `brightness
c = 255*f#
ink rgb(c,c,c),0
dot interx+1, y
interx = interx + gradient#
next y
endif
endfunction
function fract#(x as float)
a# = x - int(x)
endfunction a#
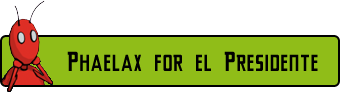