Could you specify 2D or 3D. The priciple's sorta the same: automatically, basic boxes exist for both (2D being the 4 corners of the image). The easiest way (and it uses no real resources) is to create an invisible object. On my mobile right now so can't type code but you just MAKE OBJECT BOX dummy, xsize, ysize, zsize and then update its position to that of your target object (that you are making the box for). In 2D you can just CLONE SPRITE your target object, SIZE SPRITE your clone and then HIDE SPRITE your clone. As with 3D, just add a line to reposition your hidden dummy. Beware: with 2D coords aren't centered, they're from the top left corner so you will have to calculate the size difference for repositioning. This should be enough. Will be back later and post code once you specify if it is 2D or 3D you are after (if needed).
EDIT: I shall dump some examples here, watch this space:
2D Example 1: A basic box (Seldom necessary as DBPro's built in collision is fine for square sprites)
remstart
nonZero's cheap little 2D bounding box (collision box) example.
Uses: Event areas on maps
Attack areas (tile maps, ie retro strategy games)
Bounding coxes for cursors
remend
`Setup display
SET DISPLAY MODE DESKTOP WIDTH(), DESKTOP HEIGHT(), 32
SET WINDOW ON
SET WINDOW LAYOUT 0,0,0
SYNC RATE 60
`Make Boxes and grab images for example sprites
INK RGB(240,0,0), RGB(0,0,0)
BOX 0, 0, 40, 40
GET IMAGE 1, 0, 0, 40, 40
CLS
INK RGB(0,0,240), RGB(0,0,0)
BOX 0, 0, 80, 80
INK RGB(0,0,0), RGB(0,0,0)
BOX 2, 2, 78, 78
GET IMAGE 2, 0, 0, 80, 80
CLS
INK RGB(0,240,0), RGB(0,0,0)
BOX 0, 0, 40, 40
GET IMAGE 3, 0, 0, 40, 40
`Initiate 3D backdrop and colour it black
BACKDROP ON: COLOR BACKDROP 0x000000
`Initialise the sprites (If you load them from a file using CREATE ANIMATED SPRITE, you don't need this step)
SPRITE 1, 0, 0, 1
SPRITE 2, 0, 0, 2
`I'm positioning the green box in its actual place since we don't move it again
SPRITE 3, SCREEN WIDTH() - 200, SCREEN HEIGHT() - 200, 3
`Ensure the redbox is on top
SET SPRITE PRIORITY 1, 99
`Set the ink colour to white so that text messages will display nicely
INK RGB(255,255,255), RGB(0,0,0)
`Main Loop
DO
`Position the red box (player) according to the mouse
SPRITE 1, MOUSEX(), MOUSEY(), 1
`Position the bluebox (collision box) to so it is centered around the red one (This can be done quicker, however
`I'm writing it out the long way to make it more understandable).
`Get the center of the two sprites (This should be done outside the loop to save resources - assuming sprite sizes are static)
halfbluex = SPRITE WIDTH(2) / 2
halfbluey = SPRITE HEIGHT(2) / 2
halfredx = SPRITE WIDTH(1) / 2
halfredy = SPRITE HEIGHT(1) / 2
`Then calculate the difference to give us our offset
diffx = halfbluex - halfredx
diffy = halfbluey - halfredy
`Now place the blue box (collision box) according to the offset. We use the redbox as an anchor point.
SPRITE 2, SPRITE X(1) - diffx, SPRITE Y(1) - diffy, 2
`Now we just check for collision
IF SPRITE COLLISION(2, 3) = 1: TEXT 1, 120, "It's colliding!!! Nooooooooooooo!!!!!!!! ... Told you I'd yell.": ENDIF
`Show a message:
TEXT 1, 20, "Move the mouse to move the box(es)"
TEXT 1, 40, "Press h to hide the collision box"
TEXT 1, 60, "Press s to show the collision box"
TEXT 1, 80, "Press q to quit"
TEXT 1, 100, "If you collide with th green box, I'll yell at you."
`Check key inputs
IF KEYSTATE(16) = 1: END: ENDIF
IF KEYSTATE(35) = 1: HIDE SPRITE 2: ENDIF
IF KEYSTATE(31) = 1: SHOW SPRITE 2: ENDIF
LOOP
END ` <--------- Not necessary in this case but good practice to always END with END - especially if you have functions below.
Example 2: Some basic 3D collision
remstart
nonZero's cheap little 3D bounding box (collision box) example.
Uses: Collision areas with oddly-shaped models
Improving collision by reducing the collision-area detected for.
Fast collision "Event areas" on maps (esp rugged terrain)
Notes: You can use SPHERES and any other primitive for collision too if you find DBPro's object-collision
is not meeting your needs.
remend
`Setup Varibles
GLOBAL velocity# = 0.05
GLOBAL selobj = 2
DIM objtxt$(2): objtxt$(1) = "THE CENTER OBJECT": objtxt$(2) = "THE COLLISION BOX"
`Setup display
SET DISPLAY MODE DESKTOP WIDTH(), DESKTOP HEIGHT(), 32
SET WINDOW ON
SET WINDOW LAYOUT 0,0,0
SYNC RATE 60
AUTOCAM OFF
POSITION CAMERA 0, 0,-1.5,-1
ROTATE CAMERA 0, 0,0,0
`Make our objects
`Try changing objects 1 & 2 from MAKE OBJECT CUBE to MAKE OBJECT SPHERE
MAKE OBJECT CUBE 1, 1
MAKE OBJECT CUBE 2, 2
MAKE OBJECT BOX 3, 20, 0.5, 20
`Colour objects
COLOR OBJECT 1, RGB(1,1,1)
COLOR OBJECT 2, RGB(10,250,10): GHOST OBJECT ON 2
COLOR OBJECT 3, RGB(250,10,10)
`Position objects
POSITION OBJECT 3, 0, -2, 10
POSITION OBJECT 1, 0, 2, 5
`Get offsets
offsetx = (OBJECT SIZE X(2) / 2) - (OBJECT SIZE X(1) / 2)
offsety = (OBJECT SIZE Y(2) / 2) - (OBJECT SIZE Y(1) / 2)
offsetz = (OBJECT SIZE Z(2) / 2) - (OBJECT SIZE Z(1) / 2)
`Main Loop
DO
`Check if the object bounced high enough to bring it down.
IF OBJECT POSITION Y(selobj) > 2: velocity# = -1 * velocity#: ENDIF
`Check for collision between the ground (3) and the selected object. "selobj" refers to the currently selected object
`which is either 1 or 2 depending if we're doing dollision for the object or its collision box.
IF OBJECT COLLISION(selobj, 3) = 1: : velocity# = ABS(velocity#): ENDIF
POSITION OBJECT 1, OBJECT POSITION X(1), OBJECT POSITION Y(1) + velocity#, OBJECT POSITION Z(1)
POSITION OBJECT 2, OBJECT POSITION X(1) + offsetx, OBJECT POSITION Y(1) + offsety, OBJECT POSITION Z(1) + offsetz
`Print message to screen
TEXT 1, 20, "The semi-transparent box is a homemade collision box."
TEXT 1, 40, "The center box the target-item."
TEXT 1, 60, "Press 1 to use the CENTER object for collision."
TEXT 1, 80, "Press 2 to use the COLLISION box for collision"
TEXT 1, 100, "Press h to hide the collision box."
TEXT 1, 120, "Press s to show the collission box."
TEXT 1, 140, "Press q to quit."
TEXT 1, 180, "You are currently using " + objtxt$(selobj) + " for collision"
`Check keys
IF KEYSTATE(2) = 1: selobj = 1: ENDIF
IF KEYSTATE(3) = 1: selobj = 2: ENDIF
IF KEYSTATE(35) = 1: HIDE OBJECT 2: ENDIF
IF KEYSTATE(31) = 1: SHOW OBJECT 2: ENDIF
IF KEYSTATE(16) = 1: END: ENDIF
LOOP
END ` <--------- Not necessary in this case but good practice to always END with END - especially if you have functions below.
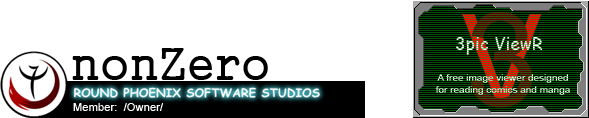