Game Performance 2.0 + Timers 2.0
With version 2.0 every bug has been fixed. The one GIDustin posted has been fixed. Everything is running almost perfectly. The seconds, milliseconds, and hours all return accurate values. Even the day which is a very small number returns correctly. Everything has been fixed to account for really small and really big numbers for timer values. The performance runs at 30 fps if you specify 30 LPS like it is suppose to. Everything has been updated!
I created two plugins. One is a timing plugin for getting back really accurate time in Mili-Seconds, Seconds, Minutes, Hours, and Days. You can also set a factor to set a 24 hour day to happen in only 2 hours of game play. The other plugin for Game Performance will increase your performance at it's maximum. It does this by returning the time back to the CPU that the application isn't using. You can also create loops at different rates.
Short version of what it can do:
- Returns the Maximum CPU time back to the CPU so you can get it to run close to 0 for usage.
- Run certain sections of code at a certain rate. (LPS = Loops Per Second) Works great for Do Loop and For Loops that only need to be updated so often.
- Works great for multi-player video games! Control how fast you send packets to the server/to the clients.
- Replaces the sync which doesn't account for performance! To run at 30 FPS just run at 30 LPS
More Benefits of my performance plugin:
Instead of simply running something in a Do Loop you can set the rate it is executed. This way you can find the minimum amount of times per second it can run. This way you cut a lot of needless calculations per second out of the equation! It is great for For Loop, While Loops, and repeat Until Loop because it can be run at any rate! You can save on performance by using these practices.
Another one of these practices is to run the display loop at the FPS you want. Every other Loop can be run at different rates.
Edit: You must set the display loop for a factor of 3 times the LPS to get a target FPS.
All display commands should be run in a loop setup like this:
Rem Project: Performance
Rem Created: Friday, July 13, 2012
Rem ***** Main Source File *****
Sync On : Sync Rate 0
GP Setup
Index = GP Create Task ("MainLoop", 60)
Index2 = GP Create Task("DisplayLoop", 30 * 3)
Do
GP Update
Loop
Function MainLoop()
` Put anything in here from math to updating something
EndFunction
Function DisplayLoop()
` Put anything that has to do with updating the display
` This includes camera commands and anything that has to do with the display
Print Screen FPS()
FastSync
EndFunction
` Dependency function
Function Dependency()
x = Object Exist(1) `Include Basic3D
x = Sprite Exist(1) `Include
x = Particles Exist(1) `Include
x = Vector Exist(1) `Include
x = Memblock Exist(1) `Include
x = Camera Exist(1) `Include
x = Image Exist(1) `Include
x = Effect Exist(1) `Include
x = Sound Exist(1) `Include
x = Mesh Exist(1) `Include
x = Music Exist(1) `Include
x = File Exist("") `Include
x = Matrix Exist(1) `Include
x = Dll Exist(1) `Include
x = Timer()
Call Function Name ""
Wait 1
Nice Wait 1
EndFunction
Instead of running the display at the maximum rate you can set the display to only run for 60 loops or 60 frames because FastSync will update each frame.
I calculate when the wait command should be run to return the
maximum time to the CPU. If you just use the wait command you are stealing from the simulation/loop time to give to the CPU.
My performance plugin doesn't
steal from the loop time and runs it at a certain rate
and returns the CPU time back to the CPU.
My commands are simple to use for any beginner. Everything is explained and shown in demos so you understand how it works. Although there is only two commands they are powerful and very useful for reducing the times a certain set of code is run and increasing performance.
Check out this image to see the CPU usage difference:
Download the demo in the next post and run Performance and No Performance programs to compare the CPU usage.
Copyright: www.myfreecopyright.com
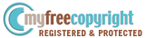
MCN:CBSE7-MU8XE-WSRDR
Just to give credit where credit is due:
- I am using some DBP commands
- I am using Matrix1Util's
Call Function Name command.
Requirements:
- You have DBP
- You have Matrix1Util's
The plugins are explained in the help files.
Help Files
Game Performance
Game performance is a plugin for getting a loop run at a certain speed while returning the maximum time back to the processor. The loop will run at a certain LPS cap or Loops Per Second which also means Frames Per Second. The rest of that time is returned back to the CPU. DBP's sync command doesn't automatically do this but my plugin will return the CPU time back to the CPU so it is running it's fastest. This plugin was created to replace the sync command. I do call fast sync in my plugin just to make sure everything is still updated correctly.
Side Note: Keep in mind these loops are not run in parallel. My multi-threading plugin will be used for this task.
Instead of simply running something in a Do Loop you can set the rate it is executed. This way you can find the minimum amount of times per second it can run. This way you cut a lot of needless calculations per second out of the equation! It is great for For Loop, While Loops, and repeat Until Loop because it can be run at any rate! You can save on performance by using these practices.
All display commands should be run in a loop setup like this:
GP Setup
Index = GP Create Task("DisplayLoop", 60) ` 60 FPS because of FastSync
Do
GP Update
Loop
Function DisplayLoop()
FastSync ` Run sync command here!
EndFunction
Instead of running the display at the maximum rate you can set the display to only run for 60 loops or 60 frames because FastSync will update each frame.
Side Note: Keep in mind these loops are not run in parallel. My multi-threading plugin will be used for this task.
` Sets up the Game Performance Plugin
GP Setup
` Input the functions name which will be representing the loop. The LPS cap which can also be thought of as the FPS is the second parameter.
Index = GP Create Task(FunctionName$, LPSCap)
` This command will update all of the loops that were created. It will run them at the rate that is set. This command will not use Fast Sync so it will be Thread Safe!
GP Update
` This command will return the task type. Count – Will run the loop x amount of times, Time – Will run the loop for x amount of time. Loop – Will loop indefinitely.
Index = GP Get Task Type(Index)
` This command will end the task Index
GP End Task Index
` This command will set the task to run for a certain duration.
GP Set Task To Time Index, Duration
` This command will set the task to run for a certain amount of times.
GP Set Task To Count Index, Count
` This command will set the task to loop indefinitely. By default tasks will loop indefinitely
GP Set Task To Loop Index
` This command will pause the task
GP Pause Task Index
` This command will resume the task
GP Resume Task Index
` This command will stop the task
GP Stop Task Index
Time
Timing plugin is for getting accurate time back in either Mili-Seconds, Seconds, Minutes, Hours, or Days. You can set the time factor which is how many hours equals 24 hour or 1 day. You can test if it is day or night time using Is Day and Is Night commands. The event timers will run a function after a certain duration. You can loop it which means it will run the function every duration seconds.
` This command is called to setup the Timing Plugin
Time Setup
` This command is how you create a new timer. It returns the index of the timer
Index = Time Create Timer()
` These commands will return the time in a certain unit (seconds, minutes, hours, ect). Returns the time# for the timer Index.
time# = Time Get Hours(Index)
time# = Time Get Minutes(Index)
time# = Time Get Seconds(Index)
time# = Time Get MiliSeconds(Index)
time# = Time Get Days(Index)
` This command will set the Factor# which is the amount of time in hours per 24 cycle.
Time Set Factor Index, Factor#
` These commands will return a 1 if it is Day or if it is Night and a 0 if it is not.
IsDay = Time Is Day()
IsNight = Time Is Night()
` This command will return how many timers are created
Index = Time Count()
` This command will delete a timer at Index
Time Delete Index
` This command will create an event timer. You provide a function name to call, a duration which is how long before you call the function, and a restart flag to if set to 1 will loop the event timer.
Index = Time Create Event Timer(FunctionName$, Duration#, Restart)
` This command will update all the Event Timers
Time Update Event Timers
` This command will return a 1 if the Event Timer has ended
HasEnded = Time Has Ended()
` This command will stop an Event Timer
Time Stop Event Timer Index
` This command will restart and Event Timer. If restart is set to 1 it will loop the Event Timer
Time Start Event Timer Index, Restart

Cost: $3.99!
Website to buy plugin:
http://www.wix.com/digitalfury/plugins
Buy all the plugins!: Sale Limited Time!
I will have a new sale after the release of Dark UV.
Plugins Details:
http://forum.thegamecreators.com/?m=forum_view&t=198638&b=5
http://forum.thegamecreators.com/?m=forum_view&t=198469&b=5
Full demo plugins are attached in the next post!
DigitalFury