Quote: "
if mouseclick()
cc=POINT(mousex(),mousey())
print cc
wait key
endif
"
Hi,OP, the error in your code (well, what glares at me is your SYNCing and WAIT KEY relationship. Since you put SYNC ON, you manually have to refresh the screen. So your problem is WAIT KEY is called before your next update. Consequently the PRINT cc statement will not actually show on the screen until after the WAIT KEY, meaning you'll see nothing the first iteration and you'll be 1 cycle behind visually than what you see physically - if it'll display correctly. Have a look at the following example on structuring your program more dynamically (The example only prints the colour's numeric value and not the R, G, B, or hex-value (since that example's been posted already):
`Refer to TheComet's example re colours. This just gives the colour value. This example is more about
`STRUCTURING and why your code didn't work.
`When setting SYNC to manual, call SYNC twice so that subsequent calls are correct the first iterarion
SYNC ON: SYNC RATE 60: SYNC: SYNC
`We'll use two variables (explained later)
curColour AS DWORD = 1
oldColour AS DWORD = 0
`Blit is a function to neaten the code: it draws the box.
Blit()
`Start a loop. I like while generally as conditions are eqated immediately.
`Basically, our primary loop iterates until "q" - KEYSTATE 16 - is pressed
WHILE KEYSTATE(16) = 0
`Here is the second loop: This loop is only entered if the mouse is clicked which
`saves resources.
WHILE MOUSECLICK() = 1
`We know the mouse was clicked so we assign the colour straight away
curColour = POINT(MOUSEX(), MOUSEY())
`Now we check if the current colour matches the previous colour (why I gave them 1 and 0 values on initialization)
`If the mouse is on the same colour still, this block gets skipped.
IF curColour <> oldColour
CLS `CLS is lazy but works for this purpose.
Blit() `Re-blit everything
PRINT "" `Now print the new colour value
PRINT SPACE$(30) + "Colour's numerical value: "; curColour
SYNC `Remember to refresh the screen now
oldColour = curColour `Finally, match the two variables for next comparison.
ENDIF
ENDWHILE
ENDWHILE
END
Function Blit()
PRINT "Hold the mousebutton down and drag to see the various colour values"
PRINT ""
PRINT "Press Q to quit..."
BOX 50, 50, 200, 200, RGB(255,255,255), RGB(180,180,180), RGB(100,100,100), RGB(0,0,0)
BOX 50, 250, 200, 450, RGB(255,0,0), RGB(0,255,0), RGB(0,0,255), RGB(0,255,255)
SYNC
EndFunction
Quote: "I would like to return a number representing the colour"
As you'll notice from my example, the numeric value is not much use for the human understanding of colour. I would recommend hex (because it is the most standardised way). If hex makes you uncomfy, try setting colours with the RGB() function and recieving them with RGBR(), RGBG() and RGBB().
Also, you really need to put your code in code snippets, like so:
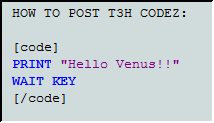
btw: you can use the "lang=" tag to specify language if you want.