No, the product ID Type parameter was set to 0.
Attached is a test project and an unsigned apk:
// Project: Amazon
// set window properties
SetWindowTitle( "TestIAP" )
inAppPurchaseSetTitle(getAppName()) // title of windows
SetWindowSize( 640, 480, 0 )
DW = GetDeviceWidth()
DH = GetDeviceHeight()
// set display properties
SetVirtualResolution( DW, DH )
SetOrientationAllowed( 0, 0, 1, 0 )
setErrorMode(2)
global glowTween as integer
global btnRed as integer
global btnGreen as integer
initIAP()
//Create the tween
glowTween = CreateTweenSprite(0.25)
SetTweenSpriteRed(glowTween,128,255,5)
SetTweenSpriteGreen(glowTween,128,255,5)
// Make the Main screem
btnRed = CreateSprite(LoadImage("\media\btnRed.jpg"))
btnGreen = CreateSprite(LoadImage("\media\btnGreen.jpg"))
SetSpritePositionByOffset(btnRed, getvirtualWidth() / 2 -100, GetVirtualHeight() / 2)
SetSpritePositionByOffset(btnGreen, getvirtualWidth() / 2 +100, GetVirtualHeight() / 2)
do
print("fps: " +str(screenfps(),1) )
print("consumable: " + str(GetInAppPurchaseAvailable(1) ))
print("entitled: " + str(GetInAppPurchaseAvailable(0) ))
//print("getAppName()" + getAppName() )
//Each cycle, check if a button was pressed
checkButtonClicks()
updateAllTweens(GetFrameTime())
Sync()
loop
end
//************************************************
function CheckButtonClicks()
//************************************************
// Checks if any buttons were pressed
// If they were, the appropriate message is constructed
// and sent to the other clients/host
//************************************************
if GetPointerReleased()
hit = GetSpriteHit(getPointerX(), getPointerY())
select hit
case btnRed
buyItem(0)
PlayTweenSprite(glowTween, btnRed,0)
endcase
case btnGreen
buyItem(1)
PlayTweenSprite(glowTween, btnGreen,0)
endcase
endselect
endif
endfunction
function initIAP()
InAppPurchaseAddProductID( "com.thegamecreators.agk_player2.agk_free_consumable", 1 )
InAppPurchaseAddProductID( "com.thegamecreators.agk_player2.agk_free_entitlement", 0 )
InAppPurchaseSetup()
endfunction
function buyItem(iPurchaseItem as integer)
iTryCount as integer
bBuySuccess as integer
inAppPurchaseActivate(iPurchaseItem)
while (getInAppPurchaseState() = 0) //this process will complete automatically. 0 for ongoing, 1 for complete (doesn't care about success or failure)
inc iTryCount
print("waiting for getInAppPurchaseState(): " + str(getInAppPurchaseState()))
print("iTryCount: " + str(iTryCount))
sync()
endwhile
bBuySuccess = getInAppPurchaseAvailable(iPurchaseItem)
endfunction bBuySuccess
1. Amazon App Tester: Log in as User 1, set all API Response Settings to 'SUCCESSFUL', delete all previous transactions.
2. Install and run testiap.apk
3. Tap the green 'Entitled' button on the right. Tap 'Get Item' in the Amazon Apps dialogue. Tap 'Close'
4. If you tap the 'Entitled' button again, the Message box 'You have already purchased that item' appears.
5. Quit and stop the app completely.
6. This Notification has appeared in the notification area:
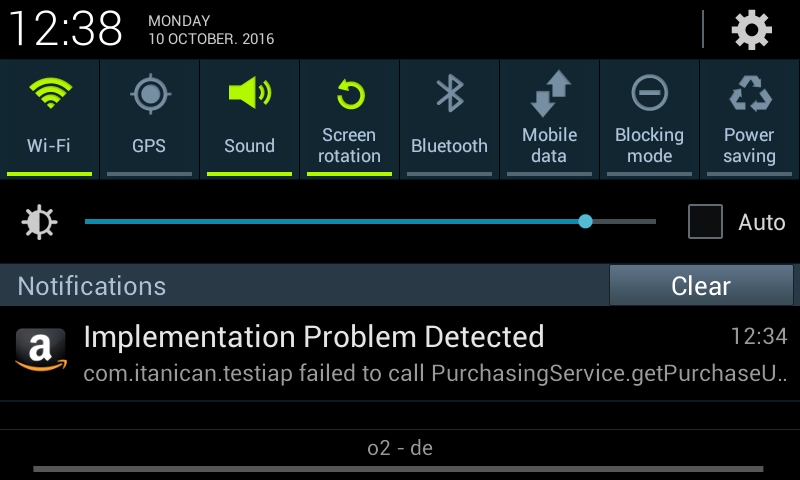
7. Run the app again. Tap the green 'Entitled' button. Tap 'Get Item' in the Amazon Apps dialogue; new dialogue box says 'You already own this item'. Tap close.
8. App hangs because GetInAppPurchaseState() does not return 1.
So the problem manifests after an Entitlement has been purchased and the app is closed and restarted. If you exit out of the loop after iTryCount has exceeded a certain number, the app cannot start a new purchase because it hasn't completed the previous transaction.
In addition, GetInAppPurchaseAvailable() on an item only returns 1 immediately after that item has been bought. InAppPurchaseRestore() does not refresh the state either.
Results of App Tester API Response Settings after an Entitlement has been purchased:
'SUCCESSFUL' - Buy dialogue opens, app hangs after tapping 'Close' in the 'You already own this item' dialogue
'FAILED' - GetInAppPurchaseState() returns 1 instantly, no hang.
'ALREADY_PURCHASED' - no dialogue boxes, GetInAppPurchaseState() remains at 0 so app hangs.