Vertical slider included now. I just wish there was some way to return a UDT from a function.
Note that I changed the UDT's a little bit.
#constant SLIDER_HORIZONTAL = 1
#constant SLIDER_VERTICAL = 2
type sliderComponent
x as integer
y as integer
min as float
max as float
value as float
isDragging as boolean
mOffsetX as float
mOffsetY as float
thumbX as float
thumbY as float
endtype
type sliderComponentUI
trackWidth as float
trackHeight as float
trackColor as dword
thumbWidth as float
thumbHeight as float
thumbColor as dword
orientation as byte
endtype
remstart
global slider as sliderComponent
slider.x = 100
slider.y = 200
slider.min = 0
slider.max = 100
slider.value = 0
remend
global s as sliderComponent
s.x = 100
s.y = 200
s.min = 0
s.max = 100
s.value = 0
s.thumbX = s.x
s.thumbY = s.y
sliderUI as sliderComponentUI
sliderUI.trackWidth = 100
sliderUI.trackHeight = 2
sliderUI.trackColor = rgb(192,192,192)
sliderUI.thumbWidth = 4
sliderUI.thumbHeight = 10
sliderUI.thumbColor = rgb(128,128,128)
sliderUI.orientation = SLIDER_VERTICAL
sync on
repeat
cls
_handleSlider(sliderUI)
set cursor 0,0
print "Value: ",s.value
print "Dragging: ",s.isDragging
sync
until spacekey()
function _handleSlider(ui as sliderComponentUI)
if ui.orientation = SLIDER_HORIZONTAL then _handleSliderHorizontal(ui)
if ui.orientation = SLIDER_VERTICAL then _handleSliderVERTICAL(ui)
endfunction
REM Horizontal Slider
function _handleSliderHorizontal(ui as sliderComponentUI)
rem draw track
ink ui.trackColor,0
y# = s.y - ui.trackHeight/2
box s.x, y#, s.x+ui.trackWidth, y#+ui.trackHeight
rem draw thumb
ink ui.thumbColor,0
y# = s.y - ui.thumbHeight/2
box s.thumbX, y#, s.thumbX+ui.thumbWidth, y#+ui.thumbHeight
rem handle mouse events
if mouseclick() = 1 and s.isDragging = 0
if mousex() >= s.thumbX and mousex() <= s.thumbX+ui.thumbWidth
if mousey() >= y# and mousey() <= y#+ui.thumbHeight
s.isDragging = 1
s.mOffsetX = s.thumbX - mousex()
endif
endif
endif
if s.isDragging = 1
s.thumbX = mousex() + s.mOffsetX
if s.thumbX < s.x then s.thumbX = s.x
if s.thumbX > (s.x+ui.trackWidth)-ui.thumbWidth then s.thumbX = (s.x+ui.trackWidth)-ui.thumbWidth
rem set slider value
s.value = ((s.max - s.min) * (s.thumbX-s.x)) / (ui.trackWidth-ui.thumbWidth)
endif
if mouseclick() <> 1 then s.isDragging = 0
endfunction
rem Vertical Slider
function _handleSliderVertical(ui as sliderComponentUI)
rem draw track
ink ui.trackColor,0
x# = s.x - ui.trackWidth/2
box x#,s.y,x#+ui.trackWidth,s.y+ui.trackHeight
rem draw thumb
ink ui.thumbColor,0
x# = s.x - ui.thumbWidth/2
box x#,s.thumbY,x#+ui.thumbWidth,s.thumbY+ui.thumbHeight
rem handle mouse events
if mouseclick() = 1 and s.isDragging = 0
if mousex() >= x# and mousex() <= x#+ui.thumbWidth
if mousey() >= s.thumbY and mousey() <= s.thumbY+ui.thumbHeight
s.isDragging = 1
s.mOffsetY = s.thumbY - mousey()
endif
endif
endif
if s.isDragging = 1
s.thumbY = mousey() + s.mOffsetY
if s.thumbY < s.y then s.thumbY = s.y
if s.thumbY > (s.y+ui.trackHeight)-ui.thumbHeight then s.thumbY = (s.y+ui.trackHeight)-ui.thumbHeight
rem set slider value
s.value = ((s.max - s.min) * (s.thumbY-s.y)) / (ui.trackHeight-ui.thumbHeight)
endif
if mouseclick() <> 1 then s.isDragging = 0
endfunction
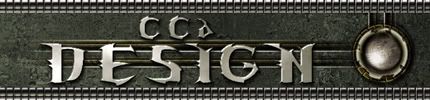