sample 3 : two swinging doors.
rem ------------------------------------------------------------------------------
rem 3D DOOR EXAMPLE
rem Method 3
rem Twin doors with a distance function
rem ------------------------------------------------------------------------------
rem temp setup
sync rate 60
sync on
rem make a green matrix for a reference
ink rgb(55,125,55),1
box 0,0,32,32
get image 1,0,0,32,32,1
make matrix 1,100,100,10,10
position matrix 1,-50,0,-50
prepare matrix texture 1,1,1,1
update matrix 1
rem temp players object
make object cube 1,2
position object 1,0,0,0
scale object 1,80,80,80
rem create some walls
cls
ink rgb(192,192,192),1
box 0,0,32,32
ink rgb(112,112,112),1
box 4,4,28,28
get image 2,0,0,32,32,1
cls
for i = 2 to 6
make object cube i,5
texture object i,2
next i
rem north wall
position object 2,0,0,12.5
scale object 2,500,100,100
rem west wall
position object 3,-15,0,0
scale object 3,100,100,600
rem east wall
position object 4,15,0,0
scale object 4,100,100,600
rem doorwall 1
position object 5,-10,0,-12.5
scale object 5,105,100,100
rem doorwall 2
position object 6,10,0,-12.5
scale object 6,105,100,100
rem create the right door
cls
ink rgb(92,92,192),1
box 0,0,32,32
ink rgb(12,12,62),1
box 4,4,28,28
get image 7,0,0,32,32,1
cls
make object cube 7,5
position object 7,0,0,0
scale object 7,130,80,10
texture object 7,7
rem create the door hinge
make object cube 8,1
position object 8,7,2,-12.5
rem make a temp mesh 35000 to create a limb for the door
make object cube 35000,1
position object 35000,7,0,-12.5
make mesh from object 1,35000
delete object 35000
rem make the right door limb
add limb 8,1,1
offset limb 8,1,-3.5,0,0
hide limb 8,1
rem glue our primitive door model to the door limb
glue object to limb 7,8,1
rem create the left door
cls
ink rgb(92,92,192),1
box 0,0,32,32
ink rgb(12,12,62),1
box 4,4,28,28
get image 9,0,0,32,32,1
cls
make object cube 9,5
position object 9,0,0,0
scale object 9,130,80,10
texture object 9,9
rem create the door hinge
make object cube 10,1
position object 10,-7,2,-12.5
rem make a temp mesh 35000 to create a limb for the door
make object cube 35000,1
position object 35000,-7,0,12.5
make mesh from object 1,35000
delete object 35000
rem make the left door limb
add limb 10,1,1
offset limb 10,1,3.5,0,0
hide limb 10,1
rem glue our primitive door model to the door limb
glue object to limb 9,10,1
rem set up the camera
position camera 0,10,-30
point camera 0,0,0
rem pre main loop
disable escapekey
ink rgb(255,255,255),1
rem start the main loop
while escapekey()=0
rem simple control of the player object
if upkey()=1 then move object 1,0.5
if downkey()=1 then move object 1,-0.5
if leftkey() = 1 then turn object left 1,2
if rightkey() = 1 then turn object right 1,2
rem mouse view
if mouseclick()=1 then cf#=1
if mouseclick()=2 then cf#=-1
cx#=wrapvalue(cx#+mousemovey()*0.5)
cy#=wrapvalue(cy#+mousemovex()*0.5)
if cx#<=290 and cx#>180 then cx#=290
if cx#>=70 and cx#<=180 then cx#=70
acx#=curveangle(cx#,acx#,2.1)
acy#=curveangle(cy#,acy#,2.1)
rotate camera acx#,acy#,0
acf#=curvevalue(cf#,acf#,20)
move camera acf#
cf#=0
move object 1,pmz#
rem the collision checking
px=object position x(1)
py=object position y(1)
pz=object position z(1)
ox=object position x(8)
oy=object position y(8)
oz=object position z(8)
dsqr = sqrdist(px,py,pz,ox,oy,oz)
if dsqr < 17
doory#=doory#+1
if doory#> 90 then doory#=90
rotate object 8,0,doory#,0
rotate object 10,0,-doory#,0
else
doory#=doory#-1
if doory#<1 then doory#=1
rotate object 8,0,doory#,0
rotate object 10,0,-doory#,0
endif
center text screen width()/2,screen height()-20,"3D Door Example - Method 3"
center text screen width()/2,screen height()-40,"distance:"+STR$(dsqr)
sync
endwhile
delete matrix 1
for i = 1 to 7
if object exist(i)
delete object i
endif
next i
end
function sqrdist(x2,y2,z2,x1,y1,z1)
dx = (x2-x1)
dy = (y2-y1)
dz = (z2-z1)
distance=sqrt(dx*dx + dy*dy + dz*dz)
endfunction distance
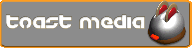