I would like to start a list of useful snippets for the AppGameKit site. If you have some ideas for small examples of AppGameKit functionality you would like to see then please post them here and I will try to post 2-3 examples per week (here as well as on the AppGameKit site).
The intention is that some of the more popular questions can be answered by looking on the AppGameKit site and also it will provide an overview of how simple some features of AppGameKit are to use in practice.
So, what would you like to see a small example of? I will do my best to create examples of as many of the suggestions posted as possible.
I should probably say I'm talking Tier 1 snippets mainly but I may be able to help with the odd Tier 2 snippet too.
Snippet list:

Choose Image from device
rem First open the image select screen
ok = ShowChooseImageScreen()
if ok = 1
rem now wait for the image to be chosen
while IsChoosingImage() = 1
sync()
endwhile
rem get the image but check that one was chosen
imageID = GetChosenImage()
if imageID = 0
message( "No image chosen" )
else
message( "Image ID: " + str(imageID) )
endif
endif

Capture image from camera
rem First open the device camera
ok = ShowImageCaptureScreen()
if ok = 1
rem now wait for the image to be captured
while IsCapturingImage() = 1
sync()
endwhile
rem get the image but check that one was captured
imageID = GetCapturedImage()
if imageID = 0
message( "No image captured" )
else
message( "Image ID: " + str(imageID) )
endif
endif

Facebook login
rem first pass your app ID from facebook
`Facebook commands are currently only
`supported on iOS
`(example ID given below)
FacebookSetup( "123456789012345" )
rem then log in
FacebookLogin()
rem check that the user has logged in
`check for a timeout in case there is
`a network problem
timeout# = timer() + 5.0
repeat
print( "Logging in" )
sync()
until GetFacebookLoggedIn() > 0 or timer() > timeout#
rem check for successful login
if GetFacebookLoggedIn() <= 0
rem display error message
message( "Could not log in to Facebook!" )
else
rem display connected message
message( "Connected to Facebook!" )
endif

Advert commands
rem set up advert provider using your app ID
`admob is currently only available on iOS
`example of Admob ID code given is not real
myAdmobID$ = "a111a111111111a"
SetAdMobDetails ( myAdmobID$ )
rem or if using inneractive use a similar setup
myInneractiveID$ = "a111a111111111a"
SetInneractiveDetails ( myInneractiveID$ )
rem display the advert
` 0=left, 1=center, 2=right
horz = 1
` 0=top, 1=center, 2=bottom
vert = 2
`test adverts can be used to ensure an ad is
`served as sometimes no advert is sent by
`the provider 1=test ad, 0=real ad
test = 1
CreateAdvert( 0, horz, vert, test )
rem occasionally you might want to refresh the
`advert so the same advert is not constantly shown
RequestAdvertRefresh()
rem to show or hide an advert use this command
`0=hidden, 1=shown
SetAdvertVisible ( 1 )
rem or to delete it entirely use this command
DeleteAdvert()

Using particles
rem Landscape App
SetDisplayAspect( 4.0/3.0 )
rem create a particle emitter
particlesID = CreateParticles( 50, 50 )
rem set the emitter creating particles
`freq is the number of particles created per second
freq = 50
size# = 2.0
SetParticlesFrequency( particlesID, freq )
SetParticlesSize( particlesID, size# )
rem set particles to fade out over 3 seconds from red to blue
SetParticlesLife( particlesID, 3.0 )
`Start color
red = 255 : green = 0 : blue = 0 : alpha = 255
AddParticlesColorKeyFrame( particlesID, 0.0, red, green, blue, alpha )
`Finish color
red = 0 : green = 0 : blue = 255 : alpha = 0
AddParticlesColorKeyFrame( particlesID, 3.0, red, green, blue, alpha )
rem Main game loop
do
rem set to the mouse / touch position
setParticlesPosition( particlesID, getPointerX(), getPointerY() )
rem update the emitter using the length
`of the previous frame
UpdateParticles( particlesID, getFrameTime() )
rem update and render the scene
Sync()
loop

Date / Time
rem print time data to screen
print("Date: "+GetCurrentDate())
day = GetDayOfWeek()
select day
case 0 : dayAsString$ = "Sunday" : endcase
case 1 : dayAsString$ = "Monday" : endcase
case 2 : dayAsString$ = "Tuesday" : endcase
case 3 : dayAsString$ = "Wednesday" : endcase
case 4 : dayAsString$ = "Thursday" : endcase
case 5 : dayAsString$ = "Friday" : endcase
case 6 : dayAsString$ = "Saturday" : endcase
endselect
print("Day : "+dayAsString$)
print("Time: "+GetCurrentTime())

Hyperlink text
rem Landscape App
SetDisplayAspect( 4.0/3.0 )
rem create a string containing the URL
url$ = "http://www.thegamecreators.com/"
rem create a text object
textID = createText( url$ )
rem Main game loop
do
rem wait for a click / tap event
if getPointerPressed()>0
rem now check if the text is under the click event
if getTextHitTest( textID, getPointerX(), getPointerY() ) > 0
rem open the browser using the url text string
openBrowser( getTextString( textID ) )
endif
endif
rem update and render
Sync()
loop

Back Button on Android
rem detecting when the back button has been pressed
rem is as simple as this!
if getRawKeyPressed(27)>0
message("Back button pressed!")
endif

Point Sprite at mouse
rem Landscape App
SetVirtualResolution( getDeviceWidth(), getDeviceHeight() )
rem create a sprite
spr = createSprite( 0 )
setSpriteSize( spr, 10, 50 )
setSpriteOffset( spr, 5, 50 )
rem position in the screen centre
centreX = getDeviceWidth()/2
centreY = getDeviceHeight()/2
setSpritePositionByOffset( spr, centreX, centreY )
rem Main game loop
do
rem get mouse pointer position
px# = getPointerX()
py# = getPointerY()
rem calculate angle between mouse and sprite
dx# = px# - centreX
dy# = py# - centreY
angle# = atanfull( dx#, dy# )
rem set the sprite angle
setSpriteAngle( spr, angle# )
rem update and render
Sync()
loop

Create a box2D sprite
rem create a sprite
` using loadSprite saves the need for loading
` the image seperately using loadImage
spr = loadSprite( "ball.png" )
rem set the size 10 percent of screen width by
` "-1" which sets the height to the correct
` size without stretching the image
setSpriteSize( spr, 10, -1)
rem initiate physics for the sprite using a value
` of 2 makes this a "dynamic" sprite which reacts
` to forces and other objects
setSpritePhysicsOn( spr, 2 )
rem make the sprite bouncy like a rubber ball
` a value of 0.0 to 1.0 is required where 1.0
` would be extremely bouncy
setSpritePhysicsRestitution( spr, 0.8 )
rem finally set the shape of the ball to circular
` 1 = circular, 2 = box, 3 = polygon (automatically created)
setSpriteShape( spr, 1 )

Add virtual joystick and button
rem 'Virtual' controls can be used on touchscreen
` devices and tested using the mouse
rem add a joystick to the bottom left of the screen
index = 1
x = 15
y = 85
size = 12
AddVirtualJoystick( index, x, y, size )
rem add a button to the bottom right of the screen
index = 1
x = 85
y = 85
size = 10
AddVirtualButton( index, x, y, size )

Ultrabook sensors
rem get ambient light level
if GetLightSensorExists()>0
print( "Ambient Light: " + str( GetRawLightLevel() ) )
endif
rem get geolocation data
if GetGeolocationExists()>0
print( "Geolocation" )
print( "City : " + GetRawGeoCity() )
print( "State : " + GetRawGeoState() )
print( "Country : " + GetRawGeoCountry() )
print( "Postcode : " + GetRawGeoPostalCode() )
print( "Latitude : " + str( GetRawGeoLatitude() ) )
print( "Longitude: " + str( GetRawGeoLongitude() ) )
endif
rem get NFC data
if GetNFCExists()>0
count = GetRawNFCCount()
if count>0
print( "NFC devices" )
endif
id = GetRawFirstNFCDevice()
while id>0
print( "Device " + GetRawNFCName( id ) + " is in range" )
if GetRawNFCDataState( id )>0
message( "Recieved data: " + GetRawNFCData( id ) )
endif
id = GetRawNextNFCDevice()
endwhile
endif
rem get Gyrometer data
if GetGyrometerExists()>0
print( "Gyrometer" )
print( "Gyro X : " + str( GetRawGyroVelocityX() ) )
print( "Gyro Y : " + str( GetRawGyroVelocityY() ) )
print( "Gyro Z : " + str( GetRawGyroVelocityZ() ) )
endif
rem get inclinometer data
if GetInclinometerExists()>0
print( "Inclinometer" )
print( "Pitch : " + str( GetRawInclinoPitch() ) )
print( "Roll : " + str( GetRawInclinoRoll() ) )
print( "Yaw : " + str( GetRawInclinoYaw() ) )
endif
rem get Orientation
if GetOrientationSensorExists()>0
print( "Orientation" )
print( "W : " + str( GetRawOrientationW() ) )
print( "X : " + str( GetRawOrientationX() ) )
print( "Y : " + str( GetRawOrientationY() ) )
print( "Z : " + str( GetRawOrientationZ() ) )
endif
Examples from community:
Accelerometer control
HTTP connect and response
View Benchmark data
3D Turret firing
Requests:

Passing files across HTTP
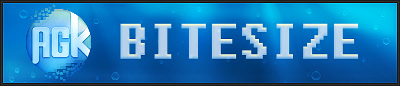
this.mess = abs(sin(times#))