Ah-ha!
Sometimes the answer is very, very simple. I simply had to re-draw the boxes that form the taskbar before using the
CLS command.
I changed the menu subroutine from this:
Rem -----------------------------------
Rem Go menu procedure
_GoMenu:
Rem Show the Go Menu
Ink RGB(0,95,255),0
x1=1 : y1=25 : x2=350 : y2=450
Box x1,y1,x2,y2
Rem Put items in the Go Menu
Ink RGB(0,125,255),0
x1=10 : y1=30 : x2=135 : y2=440
Box x1,y1,x2,y2
Rem Show the applications sub-menu
Ink RGB(0,0,0),0
Text 15,35,"Applications"
Ink RGB(255,255,255),0
Text 14,34,"Applications"
Rem Show the documents
Ink RGB(0,0,0),0
Text 15,65,"Documents"
Ink RGB(255,255,255),0
Text 14,64,"Documents"
Rem Show the computer resources
Ink RGB(0,0,0),0
Text 15,95,"The Computer"
Ink RGB(255,255,255),0
Text 14,94,"The Computer"
Rem Show the games section
Ink RGB(0,0,0),0
Text 15,125,"Games"
Ink RGB(255,255,255),0
Text 14,124,"Games"
Rem Set variables to use to check for the mouse hovering on an icon
App=0 : Docs=0 : Comp=0 : Games=0
Rem Wait until the mouse clicks again
Do
Rem Exit loop is right mouse button is pressed
If MouseClick()=2 Then Exit
Rem Check to see if the mouse is hovering over anything
Mx=MouseX() : My=MouseY() : Mc=MouseClick()
If Mx=< 135 and My=< 45 And Mx=>10 And My=> 30
App=1
Else
App=0
Endif
If Mc=1 And App=1 Then GoMenu=1
Rem If App=1...
If App=1
Ink RGB(255,255,255),0
Text 15,35,"Applications >"
Ink RGB(0,0,0),0
Text 14,34,"Applications >"
EndIf
Rem Reset variables if mouse is out-of-bounds
If App=1 Then App=0
Sync
Loop
Return
To this:
Rem -----------------------------------
Rem Go menu procedure
_GoMenu:
Rem Set variables to use to check for the mouse hovering on an icon
App=0 : Docs=0 : Comp=0 : Games=0
Rem Wait until the mouse clicks again
Do
Cls
Rem Use a box to paint the desktop
Ink RGB(55,55,55),0
x1=1 : y1=1 : x2=1023 : y2=767
Box x1,y1,x2,y2
Rem Draw the taskbar at the top of the screen
Ink RGB(0,95,255),0
x1=1 : y1=1 : x2=1023 : y2=21
Box x1,y1,x2,y2
Rem Draw the 'Go' button
Ink RGB(0,255,105),0
x1=1 : y1=1 : x2=45 : y2=21
Box x1,y1,x2,y2
Rem Draw the Go text
Ink RGB(0,0,0),0
Text 6,3,"Go"
Ink RGB(255,255,255),0
Text 5,2,"Go"
Rem Show the Go Menu
Ink RGB(0,95,255),0
x1=1 : y1=25 : x2=350 : y2=450
Box x1,y1,x2,y2
Rem Put items in the Go Menu
Ink RGB(0,125,255),0
x1=10 : y1=30 : x2=135 : y2=440
Box x1,y1,x2,y2
Rem Show the applications sub-menu
Ink RGB(0,0,0),0
Text 15,35,"Applications"
Ink RGB(255,255,255),0
Text 14,34,"Applications"
Rem Show the documents
Ink RGB(0,0,0),0
Text 15,65,"Documents"
Ink RGB(255,255,255),0
Text 14,64,"Documents"
Rem Show the computer resources
Ink RGB(0,0,0),0
Text 15,95,"The Computer"
Ink RGB(255,255,255),0
Text 14,94,"The Computer"
Rem Show the games section
Ink RGB(0,0,0),0
Text 15,125,"Games"
Ink RGB(255,255,255),0
Text 14,124,"Games"
Rem Exit loop is right mouse button is pressed
If MouseClick()=2 Then Exit
Rem Check to see if the mouse is hovering over anything
Mx=MouseX() : My=MouseY() : Mc=MouseClick()
If Mx=< 135 and My=< 45 And Mx=>10 And My=> 30
App=1
Else
App=0
Endif
Rem If App=1...
If App=1
Ink RGB(255,255,255),0
Text 15,35,"Applications >"
Ink RGB(0,0,0),0
Text 14,34,"Applications >"
EndIf
Rem Reset variables if mouse is out-of-bounds
If App=1 Then App=0
Sync
Loop
Return
That fixed it right there! Here's the whole code for anyone who's interested:
Remstart
---------------------------------------------------
- Yodaman Jer's OS Simulation Program | 6/24/09 -
---------------------------------------------------
- Comments: This program uses 2D graphics to -
- recreate the Windows XP look and feel..sort of. -
- The DrawBox function was written by TheComet. -
---------------------------------------------------
Remend
Rem Call the setup procedure
Gosub _Setup
Rem -------------------------------
Rem Main Program Loop
Rem -------------------------------
Rem Set the click variable to 0
click=0 : GoButton=0 : GoMenu=0
Do
Show Mouse : `Shows the cursor again on startup
Rem Use a box to paint the desktop
Ink RGB(55,55,55),0
x1=1 : y1=1 : x2=1023 : y2=767
Box x1,y1,x2,y2
Rem Draw the taskbar at the top of the screen
Ink RGB(0,95,255),0
x1=1 : y1=1 : x2=1023 : y2=21
Box x1,y1,x2,y2
Rem Draw the 'Go' button
Ink RGB(0,255,105),0
x1=1 : y1=1 : x2=45 : y2=21
Box x1,y1,x2,y2
Rem Draw the Go text
Ink RGB(0,0,0),0
Text 6,3,"Go"
Ink RGB(255,255,255),0
Text 5,2,"Go"
Rem Draw an unfilled box (written by TheComet)
Rem check if mouse is pressed
If MouseClick()=1
Rem set start positions
If click=0
startx=mousex()
starty=mousey()
click=1
EndIf
Rem get end positions
endx=mousex()
endy=mousey()
Rem draw box
empty_box(startx,starty,endx,endy,255,255,255)
Else
click=0
Endif
Rem Check to see if the go button is being pressed
Mx=MouseX() : My=MouseY() : Mc=MouseClick()
If Mx=< 45 and My=< 21 And Mx=>1 And My=> 1
GoButton=1
Else
GoButton=0
Endif
Rem If Go button is pressed then open the go menu
If GoButton=1
Rem Highlight the Go Button
Ink RGB(0,255,45),0
x1=1 : y1=1 : x2=45 : y2=21
Box x1,y1,x2,y2
Ink RGB(255,255,255),0
Text 6,3,"Go"
Ink RGB(0,0,0),0
Text 5,2,"Go"
If Mc=1 And GoButton=1 Then GoMenu=1
Rem Draw the Go Menu
If GoMenu=1
Gosub _GoMenu
Rem Reset variables if mouse is out-of-bounds
If GoButton=1 Then GoButton=0
If GoMenu=1 Then GoMenu=0
If Mc=1 Then Mc=0
EndIf
EndIf
Rem refresh screen
Sync
Rem end of main loop
loop
Rem ------------------------------------------------
Rem Subroutines (also known as Procedures)
Rem ------------------------------------------------
Rem The Setup Procedure
_Setup:
Set Display Mode 1024,768,32
Sync On : Sync Rate 60 : Cls 0
Hide Mouse : `Hides the DB Cursor
Return
Rem -----------------------------------
Rem Go menu procedure
_GoMenu:
Rem Set variables to use to check for the mouse hovering on an icon
App=0 : Docs=0 : Comp=0 : Games=0
Rem Wait until the mouse clicks again
Do
Cls
Rem Use a box to paint the desktop
Ink RGB(55,55,55),0
x1=1 : y1=1 : x2=1023 : y2=767
Box x1,y1,x2,y2
Rem Draw the taskbar at the top of the screen
Ink RGB(0,95,255),0
x1=1 : y1=1 : x2=1023 : y2=21
Box x1,y1,x2,y2
Rem Draw the 'Go' button
Ink RGB(0,255,105),0
x1=1 : y1=1 : x2=45 : y2=21
Box x1,y1,x2,y2
Rem Draw the Go text
Ink RGB(0,0,0),0
Text 6,3,"Go"
Ink RGB(255,255,255),0
Text 5,2,"Go"
Rem Show the Go Menu
Ink RGB(0,95,255),0
x1=1 : y1=25 : x2=350 : y2=450
Box x1,y1,x2,y2
Rem Put items in the Go Menu
Ink RGB(0,125,255),0
x1=10 : y1=30 : x2=135 : y2=440
Box x1,y1,x2,y2
Rem Show the applications sub-menu
Ink RGB(0,0,0),0
Text 15,35,"Applications"
Ink RGB(255,255,255),0
Text 14,34,"Applications"
Rem Show the documents
Ink RGB(0,0,0),0
Text 15,65,"Documents"
Ink RGB(255,255,255),0
Text 14,64,"Documents"
Rem Show the computer resources
Ink RGB(0,0,0),0
Text 15,95,"The Computer"
Ink RGB(255,255,255),0
Text 14,94,"The Computer"
Rem Show the games section
Ink RGB(0,0,0),0
Text 15,125,"Games"
Ink RGB(255,255,255),0
Text 14,124,"Games"
Rem Exit loop is right mouse button is pressed
If MouseClick()=2 Then Exit
Rem Check to see if the mouse is hovering over anything
Mx=MouseX() : My=MouseY() : Mc=MouseClick()
If Mx=< 135 and My=< 45 And Mx=>10 And My=> 30
App=1
Else
App=0
Endif
Rem If App=1...
If App=1
Ink RGB(255,255,255),0
Text 15,35,"Applications >"
Ink RGB(0,0,0),0
Text 14,34,"Applications >"
EndIf
Rem Reset variables if mouse is out-of-bounds
If App=1 Then App=0
Sync
Loop
Return
Rem Functions ------------------------------------------------------------
function empty_box(x1,y1,x2,y2,r,g,b)
rem set ink colour
ink rgb(r,g,b),0
rem make sure x1 and y1 are always smaller than x2 and y2
if x1>x2 then x3=x1:x1=x2:x2=x3
if y1>y2 then y3=y1:y1=y2:y2=y3
rem draw box
box x1,y1-1,x2,y1
box x2,y1,x2+1,y2
box x1,y2,x2,y2+1
box x1-1,y1,x1,y2
endfunction
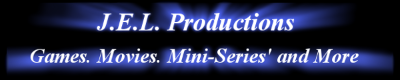
Click to go to our website!