I've been working on the PC screen settings for RTA and encountered a few things.
First off, when altering the windowed resolution the position of the window is affected by the height of the titlebar, or it doesn't take the task bar into consideration. I use a resolution that maximizes the windows height, it is placed so there's space above the window but it goes into the task bar. The size is perfect to have the window from the top of the screen down to the task bar. If the window is small enough to fit on screen and you set the resolution to the same as the startup resolution. You will notice that after setting the resolution it is positioned lower than where it was before.
Here are two snippets that shows up some inconsistencies in framerate. My laptop has a framerate of 60 but I sometimes get 70 FPS. This makes games appear choppy. It also happens more frequently in fullscreen. I have noticed this before when working on RTA but this is a more thorough test. These are commented but also print info on the screen so you can easily compare your results to mine.
Windowed
// These 3 works as intended
setsyncrate( 60, 0 )
do
print( "Syncrate 60" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "Works as intended" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setsyncrate( 120, 0 )
do
print( "Syncrate 120" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "Works as intended" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setsyncrate( 0, 0 )
do
print( "Syncrate 0" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "Works as intended" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
// These 3 works as intended
setsyncrate( 60, 0 )
setvsync( 1 )
do
print( "Syncrate 60 + V Sync ON" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "Works as intended" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setsyncrate( 120, 0 )
setvsync( 1 )
do
print( "Syncrate 120 + V Sync ON" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "Works as intended" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setsyncrate( 0, 0 )
setvsync( 1 )
do
print( "Syncrate 0 + V Sync ON" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "Works as intended" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
// Apparently setsyncrate overrides setvsync
setvsync( 1 )
setsyncrate( 60, 0 )
do
print( "VSync ON + Syncrate 60" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "SetSyncRate overrides SetVSync" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setvsync( 1 )
setsyncrate( 120, 0 )
do
print( "VSync ON + Syncrate 120" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "SetSyncRate overrides SetVSync" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setvsync( 1 )
setsyncrate( 0, 0 )
do
print( "VSync ON + Syncrate 0" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "SetSyncRate overrides SetVSync" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
// I have problems with syncrate 60 and vsync off, shouldn't this be the same as the first example?
setsyncrate( 60, 0 )
setvsync( 0 )
do
print( "Syncrate 60 + VSync OFF" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "This one gies me 70 FPS instead of 60" )
print( "Is it not the same as the first example?" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setsyncrate( 120, 0 )
setvsync( 0 )
do
print( "Syncrate 120 + VSync OFF" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "Works as intended" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setsyncrate( 0, 0 )
setvsync( 0 )
do
print( "Syncrate 0 + VSync OFF" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "Works as intended" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
Fullscreen
// Fullscreen on
SetScreenResolution( 800, 600, 1 )
// Why do I get 70 FPS at syncrate 60? This worked fine windowed
setsyncrate( 60, 0 )
do
print( "Syncrate 60" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "This gives me 70 FPS!" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setsyncrate( 120, 0 )
do
print( "Syncrate 120" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "Works as intended" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setsyncrate( 0, 0 )
do
print( "Syncrate 0" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "Works as intended" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
// All 3 give me 70 FPS when vsync is on, I got 60 in windowed
setsyncrate( 60, 0 )
setvsync( 1 )
do
print( "Syncrate 60 + V Sync ON" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "This gives me 70 FPS!" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setsyncrate( 120, 0 )
setvsync( 1 )
do
print( "Syncrate 120 + V Sync ON" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "This gives me 70 FPS!" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setsyncrate( 0, 0 )
setvsync( 1 )
do
print( "Syncrate 0 + V Sync ON" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "This gives me 70 FPS!" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
// Again, syncrate 60 gives me 70 FPS in full screen
setvsync( 1 )
setsyncrate( 60, 0 )
do
print( "VSync ON + Syncrate 60" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "This gives me 70 FPS!" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setvsync( 1 )
setsyncrate( 120, 0 )
do
print( "VSync ON + Syncrate 120" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "SetSyncRate overrides SetVSync" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setvsync( 1 )
setsyncrate( 0, 0 )
do
print( "VSync ON + Syncrate 0" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "SetSyncRate overrides SetVSync" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
// Same as windowed, syncrate 60 + vsync off gives me 70 FPS
setsyncrate( 60, 0 )
setvsync( 0 )
do
print( "Syncrate 60 + VSync OFF" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "This gives me 70 FPS!" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setsyncrate( 120, 0 )
setvsync( 0 )
do
print( "Syncrate 120 + VSync OFF" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "Works as intended" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
setsyncrate( 0, 0 )
setvsync( 0 )
do
print( "Syncrate 0 + VSync OFF" )
print( "FPS " + str(ceil(screenfps())) )
print( "" )
print( "Works as intended" )
print( "" )
print( "Press SPACE to continue" )
sync()
if getrawkeypressed(32) then exit
loop
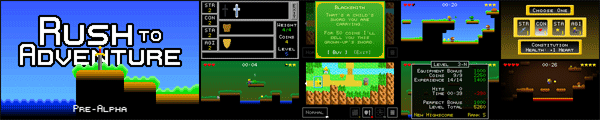