I have some draw commands. Not sure but I don't think anyone has added any of these yet. Currently it uses a temporary sprite drawing system which needs an initialisation and a single function after the sync() command in the loop to delete the temporary sprites but I'm hoping to update these functions once the "drawSprite" command is added in the next update.
Attached is a sample of the commands in use.
I may also add some further functionality to allow drawing to be done at a depth set by the user but for now these work OK:
`######## DRAW COMMANDS by Steven Holding ########
rem usage call the draw_init subroutine before
rem any other code, call the deleteDraw() function
rem after the sync() command. Other commands can
rem then be used to draw a few shapes using
rem temporary sprites
draw_init:
rem set up some draw constants
global aspect as float
aspect = getDisplayAspect()
#constant pw (1.0/getDeviceWidth())*getVirtualWidth()
#constant ph (1.0/getDeviceHeight())*getVirtualHeight()
if pw=1.0 and ph=1.0
aspect=1.0
endif
dim draw[0] as integer
draw[0] = 0
return
function deleteDraw()
num = draw[0]
for i=1 to num
deleteSprite(draw[i])
next
dim draw[0] as integer
draw[0]=0
endfunction
function drawDot(x as float, y as float, red as float, green as float, blue as float, alpha as float)
DS = createSprite(0)
setSpriteDepth(DS,0)
setSpriteSize(DS,pw,ph)
setSpriteOffset(DS,0,0)
setSpritePosition(DS,x,y)
setSpriteColor(DS,red,green,blue,alpha)
num = draw[0]+1
draw[0]=num
dim draw[num] as integer
draw[num] = DS
endfunction DS
function drawBox(x as float, y as float, angle as float, width as float, height as float, red as float, green as float, blue as float, alpha as float)
DS = createSprite(0)
setSpriteDepth(DS,0)
setSpriteSize(DS,width,height)
setSpritePosition(DS,x,y)
setSpriteAngle(DS,angle)
setSpriteColor(DS,red,green,blue,alpha)
num = draw[0]+1
draw[0]=num
dim draw[num] as integer
draw[num] = DS
endfunction DS
function drawBoxCentre(x as float, y as float, angle as float, width as float, height as float, red as float, green as float, blue as float, alpha as float)
DS = drawBox(x, y, angle, width, height, red, green, blue, alpha)
setSpriteOffset(DS,width*0.5,height*0.5)
setSpritePositionByOffset(DS,x,y)
endfunction DS
function drawLine(x1 as float, y1 as float, x2 as float, y2 as float, red as float, green as float, blue as float, alpha as float)
DS = createSprite(0)
setSpriteDepth(DS,0)
dx#=x2-x1
dy#=(y2-y1)/aspect
l# = sqrt(dx#*dx#+dy#*dy#)
setSpriteSize(DS,l#,ph)
a# = atanfull(dx#,dy#)-90.0
setSpriteAngle(DS,a#)
setSpriteOffset(DS,0,ph*0.5)
setSpritePosition(DS,x1,y1)
setSpriteColor(DS,red,green,blue,alpha)
num = draw[0]+1
draw[0]=num
dim draw[num] as integer
draw[num] = DS
endfunction DS
function drawCircle(x as float, y as float, radius as float, red as float, green as float, blue as float, alpha as float)
num=0
st# = 8.0/radius
for c#=0.0 to 360.0 step st#
num=num+1
drawDot(x+(radius*sin(c#)),y+(radius*aspect*cos(c#)),red,green,blue,alpha)
next
endfunction num
function drawFilledCircle(x as float, y as float, radius as float, red as float, green as float, blue as float, alpha as float)
num=0
sr# = radius*radius
for cx# = -radius to radius step pw
num=num+1
h# = sqrt(sr#-cx#*cx#)*aspect
drawBox(x-cx#,y-h#,0,pw,h#*2.0-pw,red,green,blue,alpha)
next
endfunction num
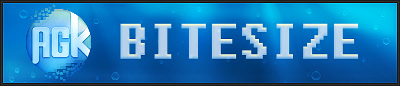